帮我用vue写一个用户中心代码
时间: 2023-06-06 16:04:18 浏览: 110
好的,我可以为您提供一些Vue的代码,以创建一个基本的用户中心页面。请注意,这是一个简单的示例,您需要根据您的具体要求进行修改和定制。
首先,您需要创建一个Vue组件来管理用户中心页面。在这个组件中,您可以使用Vue的数据绑定和计算属性来处理用户数据,并使用Vue的生命周期方法来处理页面的初始化和销毁。
以下是一个示例组件,它提供了一个用户列表和一个表格来显示每个用户的详细信息:
```html
<template>
<div class="user-center">
<h1>用户中心</h1>
<table>
<thead>
<tr>
<th>姓名</th>
<th>邮箱</th>
<th>电话</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="user in users" :key="user.id">
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
<td>{{ user.phone }}</td>
<td>
<button @click="editUser(user)">编辑</button>
<button @click="deleteUser(user)">删除</button>
</td>
</tr>
</tbody>
</table>
<div v-if="editingUser">
<h2>编辑用户</h2>
<form @submit.prevent="saveUser">
<label>
姓名:<input type="text" v-model="editingUser.name">
</label>
<label>
邮箱:<input type="email" v-model="editingUser.email">
</label>
<label>
电话:<input type="tel" v-model="editingUser.phone">
</label>
<button type="submit">保存</button>
<button type="button" @click="cancelEdit">取消</button>
</form>
</div>
</div>
</template>
<script>
export default {
data() {
return {
users: [
{ id: 1, name: '张三', email: 'zhangsan@example.com', phone: '13311112222' },
{ id: 2, name: '李四', email: 'lisi@example.com', phone: '13311113333' },
{ id: 3, name: '王五', email: 'wangwu@example.com', phone: '13311114444' },
],
editingUser: null,
}
},
computed: {
sortedUsers() {
return this.users.sort((a, b) => a.name.localeCompare(b.name))
},
},
methods: {
editUser(user) {
this.editingUser = { ...user }
},
deleteUser(user) {
this.users = this.users.filter(u => u.id !== user.id)
},
saveUser() {
const existingUserIndex = this.users.findIndex(u => u.id === this.editingUser.id)
if (existingUserIndex >= 0) {
this.users.splice(existingUserIndex, 1, this.editingUser)
} else {
this.editingUser.id = this.users.length + 1
this.users.push(this.editingUser)
}
this.cancelEdit()
},
cancelEdit() {
this.editingUser = null
},
},
created() {
console.log('用户中心页面创建!')
},
destroyed() {
console.log('用户中心页面销毁!')
},
}
</script>
<style>
table {
border-collapse: collapse;
border-spacing: 0;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
border-bottom: 1px solid #ddd;
}
th:first-child, td:first-child {
border-left: 1px solid #ddd;
}
th:last-child, td:last-child {
border-right: 1px solid #ddd;
}
button {
margin: 8px;
}
</style>
```
在这个示例中,我们首先定义了一个用户列表和一个编辑表单。然后,在Vue的`data`选项中,我们定义了一个`users`数组来保存用户数据,以及一个`editingUser`对象来保存当前正在编辑的用户。在`computed`选项中,我们定义了一个`sortedUsers`计算属性来顺序排列`users`数组中的用户。然后,在`methods`选项中,我们定义了一些方法来处理用户编辑、删除和保存。
当组件被创建时,我们使用Vue的`created`钩子函数来输出一条调试信息。当组件被销毁时,我们使用Vue的`destroyed`钩子函数来输出一条调试信息。
要将这个组件添加到您的Vue应用程序中,请按照以下步骤操作:
1. 在vue文件中导入这个组件;
2. 在Vue实例的`components`选项中注册该组件;
3. 在Vue模板中使用该组件的标签。
示例代码:
```html
<template>
<div>
<!-- 其他页面内容 -->
<user-center></user-center>
</div>
</template>
<script>
import UserCenter from './UserCenter.vue'
export default {
components: { UserCenter },
// 其他Vue配置项
}
</script>
```
这应该能够帮助您开始编写一个基本的Vue用户中心页面。请记住,这只是一个示例,您需要进一步定制和添加功能以满足您的特定要求。
阅读全文
相关推荐
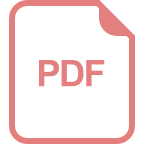
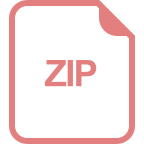
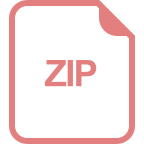
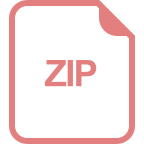
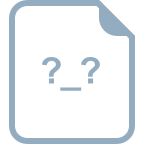
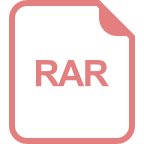
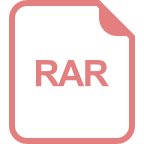
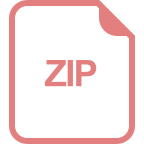
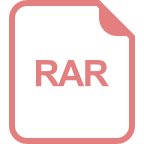
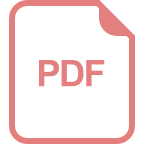
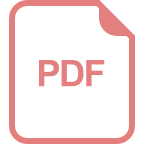
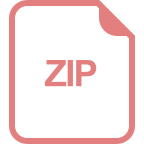
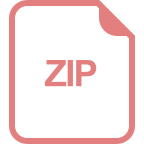
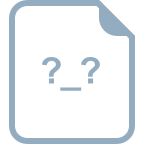
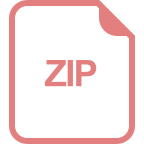
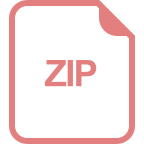
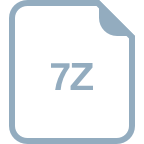
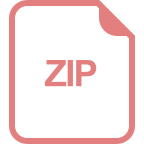
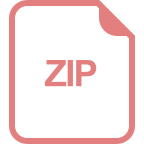