Java 怎么获取多线程的返回值
时间: 2024-03-25 13:38:23 浏览: 60
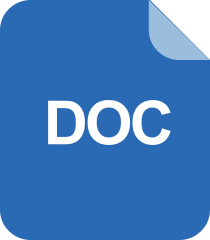
多线程 Java
在 Java 中,获取多线程的返回值可以通过以下几种方式:
1. 使用 Callable 和 Future 接口。Callable 接口定义了 call() 方法用于返回结果,Future 接口用于获取异步计算结果。示例代码如下:
```
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
public class ThreadTest {
public static void main(String[] args) throws ExecutionException, InterruptedException {
Callable<Integer> callable = new Callable<Integer>() {
@Override
public Integer call() throws Exception {
// 在这里编写耗时的业务逻辑
return 100;
}
};
FutureTask<Integer> futureTask = new FutureTask<>(callable);
Thread thread = new Thread(futureTask);
thread.start();
Integer result = futureTask.get(); // 获取异步计算结果
System.out.println(result);
}
}
```
2. 使用 Runnable 接口和共享变量。在多线程环境下,可以使用共享变量来传递和获取线程的返回结果。示例代码如下:
```
public class ThreadTest {
static int result = 0;
public static void main(String[] args) throws InterruptedException {
Runnable runnable = new Runnable() {
@Override
public void run() {
// 在这里编写耗时的业务逻辑
result = 100;
}
};
Thread thread = new Thread(runnable);
thread.start();
thread.join();
System.out.println(result);
}
}
```
以上两种方式都可以获取多线程的返回值,具体使用哪种方式取决于业务需求。
阅读全文
相关推荐
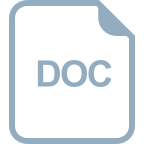
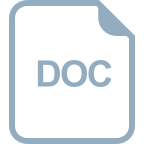
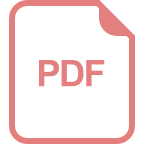

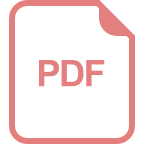
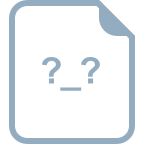
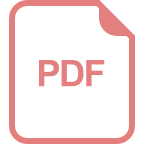
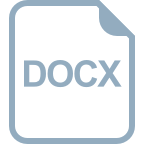
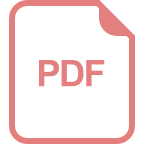
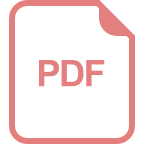
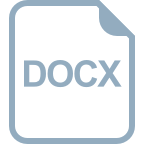
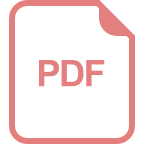
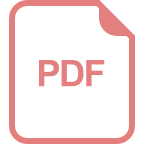
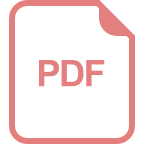



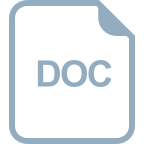