简要用java写一个学籍管理系统,要求附带学生成绩,学号,姓名,年龄,能够将指定的信息删除,可以统计全部学生的总成绩,及其在班上的排名,能够统计各科的平均成绩及及格率,要求有错误提示功能,例如性别只能输入男女,输入错误提示重新输入。
时间: 2024-05-22 11:13:01 浏览: 120
import java.util.Scanner;
public class StudentManagementSystem {
private static Student[] students;
private static int totalStudents;
private static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
System.out.println("欢迎使用学籍管理系统!");
System.out.print("请输入学生数量:");
totalStudents = scanner.nextInt();
students = new Student[totalStudents];
for (int i = 0; i < totalStudents; i++) {
System.out.println("请输入第" + (i + 1) + "个学生的信息:");
System.out.print("学号:");
int id = scanner.nextInt();
System.out.print("姓名:");
String name = scanner.next();
System.out.print("年龄:");
int age = scanner.nextInt();
System.out.print("性别(男/女):");
String gender = scanner.next();
while (!gender.equals("男") && !gender.equals("女")) {
System.out.print("性别输入错误,请重新输入(男/女):");
gender = scanner.next();
}
System.out.print("Java成绩:");
int javaScore = scanner.nextInt();
System.out.print("C++成绩:");
int cppScore = scanner.nextInt();
System.out.print("Python成绩:");
int pythonScore = scanner.nextInt();
students[i] = new Student(id, name, age, gender, javaScore, cppScore, pythonScore);
}
boolean exit = false;
while (!exit) {
System.out.println("---------------------------------------");
System.out.println("请选择需要执行的操作:");
System.out.println("1. 删除指定学生信息");
System.out.println("2. 统计全部学生总成绩及排名");
System.out.println("3. 统计各科平均成绩及及格率");
System.out.println("4. 退出系统");
System.out.print("请选择:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
deleteStudent();
break;
case 2:
calculateTotalScoreAndRank();
break;
case 3:
calculateAverageScoreAndPassRate();
break;
case 4:
exit = true;
break;
default:
System.out.println("输入错误,请重新输入!");
}
}
System.out.println("感谢使用学籍管理系统!");
}
private static void deleteStudent() {
System.out.print("请输入要删除的学生的学号:");
int id = scanner.nextInt();
int index = -1;
for (int i = 0; i < totalStudents; i++) {
if (students[i].getId() == id) {
index = i;
break;
}
}
if (index == -1) {
System.out.println("学号为" + id + "的学生不存在!");
} else {
for (int i = index; i < totalStudents - 1; i++) {
students[i] = students[i + 1];
}
students[totalStudents - 1] = null;
totalStudents--;
System.out.println("学号为" + id + "的学生信息已成功删除!");
}
}
private static void calculateTotalScoreAndRank() {
int[] totalScores = new int[totalStudents];
for (int i = 0; i < totalStudents; i++) {
totalScores[i] = students[i].getJavaScore() + students[i].getCppScore() + students[i].getPythonScore();
}
for (int i = 0; i < totalStudents - 1; i++) {
for (int j = i + 1; j < totalStudents; j++) {
if (totalScores[j] > totalScores[i]) {
int tempScore = totalScores[i];
totalScores[i] = totalScores[j];
totalScores[j] = tempScore;
Student tempStudent = students[i];
students[i] = students[j];
students[j] = tempStudent;
}
}
}
for (int i = 0; i < totalStudents; i++) {
System.out.println("第" + (i + 1) + "名:" + students[i].getName() + ",总成绩:" + totalScores[i]);
}
}
private static void calculateAverageScoreAndPassRate() {
int javaTotalScore = 0;
int cppTotalScore = 0;
int pythonTotalScore = 0;
int javaPassCount = 0;
int cppPassCount = 0;
int pythonPassCount = 0;
for (int i = 0; i < totalStudents; i++) {
javaTotalScore += students[i].getJavaScore();
cppTotalScore += students[i].getCppScore();
pythonTotalScore += students[i].getPythonScore();
if (students[i].getJavaScore() >= 60) {
javaPassCount++;
}
if (students[i].getCppScore() >= 60) {
cppPassCount++;
}
if (students[i].getPythonScore() >= 60) {
pythonPassCount++;
}
}
System.out.println("Java平均成绩:" + javaTotalScore / totalStudents);
System.out.println("C++平均成绩:" + cppTotalScore / totalStudents);
System.out.println("Python平均成绩:" + pythonTotalScore / totalStudents);
System.out.println("Java及格率:" + (double) javaPassCount / totalStudents * 100 + "%");
System.out.println("C++及格率:" + (double) cppPassCount / totalStudents * 100 + "%");
System.out.println("Python及格率:" + (double) pythonPassCount / totalStudents * 100 + "%");
}
}
class Student {
private int id;
private String name;
private int age;
private String gender;
private int javaScore;
private int cppScore;
private int pythonScore;
public Student(int id, String name, int age, String gender, int javaScore, int cppScore, int pythonScore) {
this.id = id;
this.name = name;
this.age = age;
this.gender = gender;
this.javaScore = javaScore;
this.cppScore = cppScore;
this.pythonScore = pythonScore;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getGender() {
return gender;
}
public int getJavaScore() {
return javaScore;
}
public int getCppScore() {
return cppScore;
}
public int getPythonScore() {
return pythonScore;
}
}
阅读全文
相关推荐
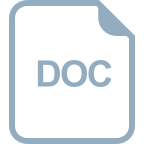
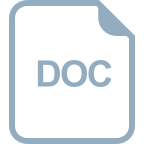
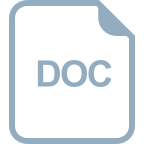

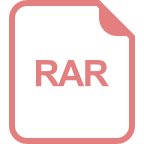
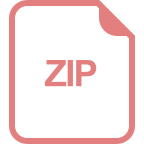
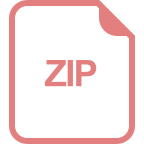
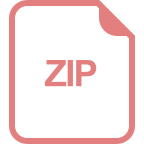
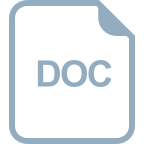
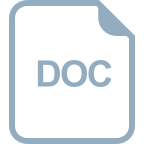
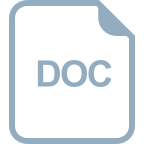
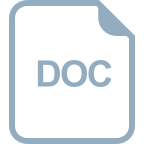
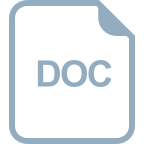





