图形用户界面课程 3:多项选择图形用户界面 编写程序以创建多项选择测验 需要一个基于 GUI 的程序,使人们能够从多项选择题库中进行测试。每个问题包括: 问题文本; 4个可能的答案; 正确答案的数量(1-4,而不是0-3); 问题按顺序保存在文本文件中,每个问题保存在 6 行上。问题文本文件中的示例条目可能如下所示: 该计划应具有以下特点: 一个包含“打开”和“退出”的菜单,其中“打开”启动 JFileChooser 以选择包含问题的文件,“退出”结束程序。 加载文件后,GUI 应一次显示一个问题及其答案。 用户应该能够选择一个答案,并且应该通知他们是否正确。 应让用户知道正确回答的数量和回答的问题总数。 用户应该只有在回答了当前问题后才能继续下一个问题。 回答完所有问题后,应通知用户他们的总分以及游戏已完成。现在应启用“打开”菜单项以启动新测验。(可选)您可以添加重新启动菜单项以重做当前测验。
时间: 2024-02-11 11:09:46 浏览: 26
好的,您的问题是如何在 Java 中实现一个包含多项选择题的 GUI 测验程序?
实现这样一个程序需要用到 Java 的 GUI 库,比如 Swing 或 JavaFX。程序需要读取题库文件并将其加载到程序中。然后,程序需要显示问题和选项,并等待用户选择答案。用户选择答案后,程序需要检查答案是否正确,并记录用户的得分。然后,程序需要显示下一个问题,重复上述过程,直到所有问题都被回答。最后,程序需要显示用户的总分并结束游戏。
以下是一个简单的 Java Swing 实现的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
public class QuizProgram extends JFrame implements ActionListener {
private JMenuBar menuBar;
private JMenu fileMenu;
private JMenuItem openMenuItem, exitMenuItem;
private JPanel questionPanel, answerPanel;
private JLabel questionLabel;
private JRadioButton[] answerButtons;
private JButton submitButton;
private int currentQuestion;
private int correctAnswers;
private ArrayList<Question> questions;
public QuizProgram() {
super("Quiz Program");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLocationRelativeTo(null);
// Create menu bar and menu items
menuBar = new JMenuBar();
fileMenu = new JMenu("File");
openMenuItem = new JMenuItem("Open");
exitMenuItem = new JMenuItem("Exit");
openMenuItem.addActionListener(this);
exitMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
fileMenu.addSeparator();
fileMenu.add(exitMenuItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// Create question and answer panels
questionPanel = new JPanel();
answerPanel = new JPanel();
questionLabel = new JLabel();
answerButtons = new JRadioButton[4];
ButtonGroup answerGroup = new ButtonGroup();
for (int i = 0; i < 4; i++) {
answerButtons[i] = new JRadioButton();
answerGroup.add(answerButtons[i]);
answerPanel.add(answerButtons[i]);
}
submitButton = new JButton("Submit");
submitButton.addActionListener(this);
// Add components to frame
questionPanel.add(questionLabel);
add(questionPanel, BorderLayout.NORTH);
add(answerPanel, BorderLayout.CENTER);
add(submitButton, BorderLayout.SOUTH);
// Initialize variables
currentQuestion = 0;
correctAnswers = 0;
questions = new ArrayList<Question>();
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == openMenuItem) {
// Show file chooser dialog and load questions
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
loadQuestions(file);
showNextQuestion();
}
} else if (e.getSource() == exitMenuItem) {
// Exit program
System.exit(0);
} else if (e.getSource() == submitButton) {
// Check answer and show next question
if (checkAnswer()) {
correctAnswers++;
}
currentQuestion++;
if (currentQuestion < questions.size()) {
showNextQuestion();
} else {
// Show final score and restart game
JOptionPane.showMessageDialog(this, "You got " + correctAnswers + " out of " + questions.size() + " correct.");
restartGame();
}
}
}
private void loadQuestions(File file) {
// Read questions from file
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(",");
String questionText = parts[0];
String[] answerTexts = Arrays.copyOfRange(parts, 1, 5);
int numCorrect = Integer.parseInt(parts[5]);
Question question = new Question(questionText, answerTexts, numCorrect);
questions.add(question);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private void showNextQuestion() {
// Show next question and reset answer buttons
Question question = questions.get(currentQuestion);
questionLabel.setText(question.getQuestionText());
String[] answerTexts = question.getAnswerTexts();
for (int i = 0; i < 4; i++) {
answerButtons[i].setText(answerTexts[i]);
answerButtons[i].setSelected(false);
}
}
private boolean checkAnswer() {
// Check if selected answer is correct
Question question = questions.get(currentQuestion);
int numCorrect = question.getNumCorrect();
int numSelected = 0;
for (int i = 0; i < 4; i++) {
if (answerButtons[i].isSelected()) {
numSelected++;
}
}
if (numSelected != numCorrect) {
return false;
}
for (int i = 0; i < 4; i++) {
if (answerButtons[i].isSelected() != question.isCorrect(i)) {
return false;
}
}
return true;
}
private void restartGame() {
// Reset game variables and show file chooser dialog
currentQuestion = 0;
correctAnswers = 0;
questions.clear();
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
loadQuestions(file);
showNextQuestion();
}
}
public static void main(String[] args) {
QuizProgram quizProgram = new QuizProgram();
quizProgram.setVisible(true);
}
}
class Question {
private String questionText;
private String[] answerTexts;
private int numCorrect;
public Question(String questionText, String[] answerTexts, int numCorrect) {
this.questionText = questionText;
this.answerTexts = answerTexts;
this.numCorrect = numCorrect;
}
public String getQuestionText() {
return questionText;
}
public String[] getAnswerTexts() {
return answerTexts;
}
public int getNumCorrect() {
return numCorrect;
}
public boolean isCorrect(int index) {
// Check if answer at index is correct
if (index < 0 || index >= 4) {
return false;
}
if (answerTexts[index].isEmpty()) {
return false;
}
return answerTexts[index].charAt(0) == 'T';
}
}
```
该程序包括一个菜单栏,其中包含“打开”和“退出”菜单项。单击“打开”将启动文件选择器,用于选择包含问题的文件。该程序可以处理保存在文本文件中的问题。每个问题占用文件中的 6 行,其中第 1 行包含问题文本,第 2-5 行包含答案选项,第 6 行包含正确答案的数量(1-4)。
当文件被加载后,程序将显示第一个问题和答案选项。用户可以选择一个答案并单击“提交”按钮。程序将检查选择的答案是否正确,如果正确,则将该问题的分数添加到总分中。程序将显示下一个问题,并等待用户选择答案。重复这个过程,直到所有问题都被回答。最后,程序将显示用户的总分并等待用户选择“打开”或“退出”菜单项。
在这个示例程序中,我们使用了一个 Question 类来存储每个问题及其答案。每个问题都由一个问题文本、四个答案选项和正确答案的数量组成。程序在加载文件时创建 Question 对象,并将它们存储在一个 ArrayList 中。程序使用 Question 对象来显示问题和答案选项,并检查用户选择的答案是否正确。
以上是一个简单的实现示例,您可以根据自己的需求进行修改和扩展。
相关推荐
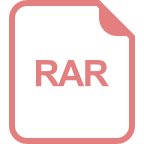
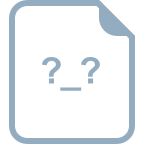
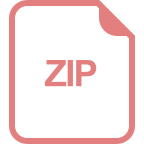














