提供一个vue3实现音频文字同步的案例
时间: 2023-07-07 16:23:45 浏览: 322
以下是一个使用 Vue3 实现音频文字同步的案例,代码如下:
```html
<template>
<div>
<audio ref="audio" src="./audio.mp3" @timeupdate="showText"></audio>
<div v-for="item in textList" :key="item.time" :style="{top: item.top + 'px'}">{{item.text}}</div>
</div>
</template>
<script>
import { ref, reactive, onMounted } from 'vue';
export default {
setup() {
const audio = ref(null);
const textList = reactive([]);
const text = ref('');
function showText() {
const currentTime = Math.floor(audio.value.currentTime);
const index = text.value.indexOf(`[${currentTime}`);
if (index !== -1) {
const endIndex = text.value.indexOf(']', index);
const time = text.value.slice(index + 1, endIndex);
const content = text.value.slice(endIndex + 1, text.value.indexOf(`[${currentTime + 1}`) !== -1 ? text.value.indexOf(`[${currentTime + 1}`) : text.value.length);
textList.push({
time: parseInt(time),
text: content,
top: textList.length * 30
});
}
}
onMounted(() => {
fetch('./audio.txt')
.then(response => response.text())
.then(data => {
text.value = data;
});
});
return {
audio,
textList,
showText
}
}
}
</script>
```
上述代码中,我们首先在模板中引入了一个 audio 标签,并且绑定了 timeupdate 事件,当音频播放时会触发该事件并执行 showText 函数。
在 setup 函数中,我们使用 ref 和 reactive 创建了一些响应式数据和函数。其中,audio 用于绑定 audio 标签,textList 用于保存文字列表,text 用于保存从文件中读取的文字内容。
showText 函数会在音频播放时被调用,它会根据当前播放时间从文字内容中获取相应的文字,并将其添加到 textList 中。在模板中,我们使用 v-for 遍历 textList 并将文字显示在界面上。
需要注意的是,上述代码中的文字内容是从文本文件中读取的,它的格式应该是每行一个时间戳和相应的文字内容,例如:
```txt
[0]Hello world!
[3]How are you?
[6]I'm fine, thank you!
```
这样,我们就可以实现一个简单的音频文字同步功能。
阅读全文
相关推荐
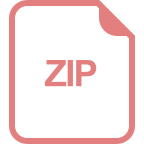
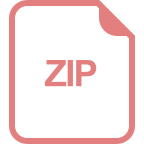
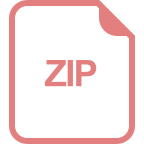















