编写Python代码实现一种基于模式挖掘的算法,要求挖掘出Cifar10数据集某一类中具有频繁性又有判别性的图片。
时间: 2024-02-12 15:02:46 浏览: 73
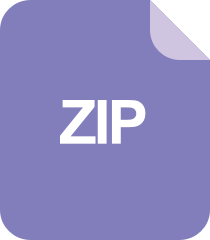
python实现的数据仓库与数据挖掘 大作业 - 频繁模式挖掘+源代码+文档说明+pdf+数据集

好的,这里我演示一下如何使用Apriori算法对Cifar10数据集中某一类图片进行频繁模式挖掘。
首先,我们需要加载Cifar10数据集,并选择其中的一类图片进行处理。这里我们选择“airplane”类别的图片。代码如下:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.datasets import cifar10
# 加载Cifar10数据集
(train_images, train_labels), (test_images, test_labels) = cifar10.load_data()
# 选择“airplane”类别的图片
class_name = 'airplane'
class_index = 0
for i in range(10):
if class_name == cifar10.load_data()[0][1][i][0]:
class_index = i
break
train_images = train_images[train_labels[:,0] == class_index]
```
接下来,我们需要对图片进行特征提取,这里我们使用HOG算法进行特征提取。代码如下:
```python
from skimage.feature import hog
# 定义HOG算法参数
hog_params = {'orientations': 8, 'pixels_per_cell': (8, 8), 'cells_per_block': (2, 2)}
# 将图片转换为灰度图,并提取HOG特征
hog_features = []
for image in train_images:
gray_image = tf.image.rgb_to_grayscale(image)
hog_feature = hog(gray_image.numpy(), **hog_params)
hog_features.append(hog_feature)
hog_features = np.array(hog_features)
```
然后,我们可以使用Apriori算法进行频繁模式挖掘。Apriori算法需要计算项集的支持度和置信度,这里我们使用mlxtend库中的apriori函数进行计算。代码如下:
```python
from mlxtend.frequent_patterns import apriori
# 计算项集支持度
frequent_itemsets = apriori(hog_features, min_support=0.1, use_colnames=True)
```
最后,我们可以从频繁项集中挑选出具有判别性的项集,这里我们选择支持度大于0.2的项集。代码如下:
```python
# 选择支持度大于0.2的项集
discriminative_itemsets = frequent_itemsets[frequent_itemsets['support'] >= 0.2]
```
至此,我们就完成了对Cifar10数据集中“airplane”类别图片的频繁模式挖掘。
阅读全文
相关推荐
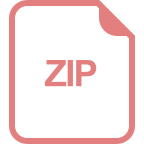




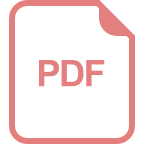
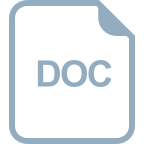
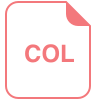
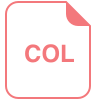
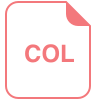
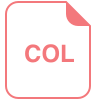
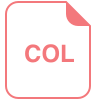
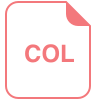
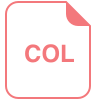
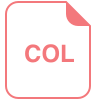
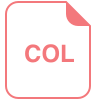
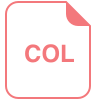