用c++语言写程序,满足如下要求编写程序,设计类,输出学生的信息并计算出学生人数和平均成绩。
时间: 2024-03-24 17:36:34 浏览: 58
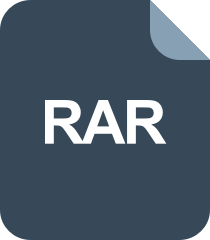
基于c++的学生成绩管理系统设计与代码实现
好的,我可以帮你回答这个问题。
以下是用C++语言编写的程序,满足你的要求:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name;
int score;
public:
Student(string name, int score) {
this->name = name;
this->score = score;
}
string getName() {
return name;
}
int getScore() {
return score;
}
};
int main() {
int n;
cout << "请输入学生人数:";
cin >> n;
Student* students = new Student[n];
int totalScore = 0;
for (int i = 0; i < n; i++) {
string name;
int score;
cout << "请输入第" << i + 1 << "个学生的姓名和成绩:";
cin >> name >> score;
students[i] = Student(name, score);
totalScore += score;
}
double averageScore = (double) totalScore / n;
cout << "学生信息如下:" << endl;
for (int i = 0; i < n; i++) {
cout << "姓名:" << students[i].getName() << ",成绩:" << students[i].getScore() << endl;
}
cout << "学生人数:" << n << endl;
cout << "平均成绩:" << averageScore << endl;
delete[] students;
return 0;
}
```
在这个程序中,我们定义了一个`Student`类来存储每个学生的姓名和成绩。在`main`函数中,我们首先询问用户输入学生人数,然后使用`new`运算符动态分配一个`Student`数组。接着,我们使用一个循环来询问每个学生的姓名和成绩,并将其存储到相应的`Student`对象中。同时,我们还累加每个学生的成绩,以便计算平均成绩。最后,我们遍历`Student`数组,输出每个学生的姓名和成绩,并输出学生人数和平均成绩。最后别忘了使用`delete[]`运算符释放内存。
希望这个程序能够帮到你,如果你还有其他问题,请随时问我。
阅读全文
相关推荐
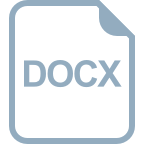
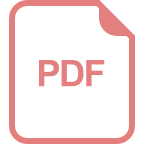
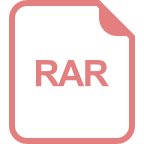
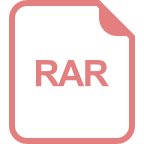
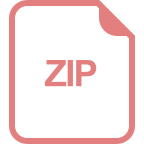
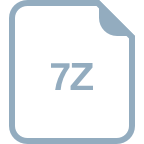
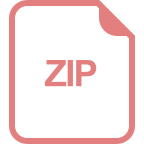
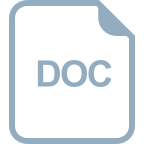
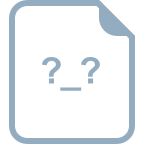
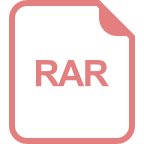
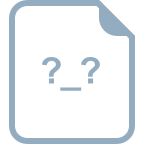
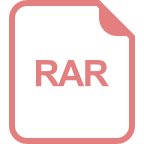
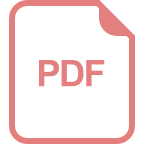
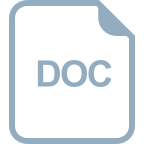
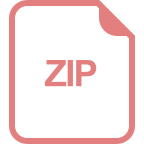