input 的校验只能输入中文和数字
时间: 2023-07-21 15:25:49 浏览: 86
你可以通过 JavaScript 的正则表达式来实现输入框只能输入中文和数字的校验。以下是一个示例代码:
```html
<input type="text" id="input" onkeyup="validateInput()">
<script>
function validateInput() {
var input = document.getElementById("input");
var regExp = /^[\u4e00-\u9fa5\d]*$/; // 匹配中文和数字
if (!regExp.test(input.value)) { // 如果输入不合法
input.value = input.value.replace(/[^\u4e00-\u9fa5\d]/g, ""); // 将非中文和数字字符替换为空
}
}
</script>
```
在这个例子中,我们为输入框添加了一个 `onkeyup` 事件,它会调用 `validateInput()` 函数。在这个函数中,我们首先获取了输入框元素,并使用正则表达式 `/^[\u4e00-\u9fa5\d]*$/` 匹配中文和数字。如果输入框中存在非中文和数字字符,我们就使用 `replace()` 方法将它们替换为空。这样,用户就只能输入中文和数字了。
相关问题
el-input 校验只能输入中文和数字
您可以使用element-ui提供的el-input组件的自定义校验规则功能来实现只能输入中文和数字的校验,具体步骤如下:
1. 在el-input组件上添加自定义校验规则属性 :validator
```
<el-input v-model="inputValue" :validator="validateInput"></el-input>
```
2. 在methods中定义校验函数 validateInput,判断输入的内容是否符合要求
```
methods: {
validateInput(rule, value, callback) {
const reg = /^[\u4e00-\u9fa5\d]+$/ // 只能输入中文和数字
if (!value) {
callback(new Error('请输入内容'))
} else if (!reg.test(value)) {
callback(new Error('只能输入中文和数字'))
} else {
callback()
}
}
}
```
这样,用户在输入框中输入内容时,只有输入中文和数字才不会出现校验错误提示。
正则校验input框只能输入数字
可以使用正则表达式来验证输入框中只能输入数字。以下是一个示例正则表达式:
```javascript
/^[0-9]*$/
```
该正则表达式可以匹配只包含数字的字符串。将其应用于input框的值,如果匹配成功,则表示输入框中只包含数字。
可以使用如下的代码来实现:
```javascript
var inputBox = document.getElementById('inputBox');
inputBox.addEventListener('input',function(){
var inputValue = inputBox.value;
if(!/^[0-9]*$/.test(inputValue)){
inputBox.value = inputValue.replace(/[^\d]/g,'');
}
});
```
此代码将监听input框的输入事件,在输入时检查输入的值是否为数字,如果不是,则使用正则表达式将其替换为纯数字字符串。
阅读全文
相关推荐
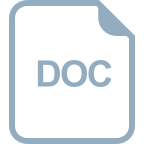
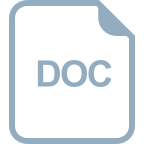
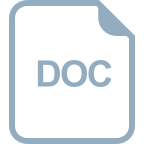













