svm二分类python实现
时间: 2023-12-04 22:28:09 浏览: 31
Sure, SVM binary classification can be implemented in Python using the scikit-learn library. It involves defining a training dataset, preprocessing the data, selecting the appropriate kernel function and parameters, and finally training the model and making predictions on test data. Is there anything specific you would like to know about this?
相关问题
svm二分类python
要在Python中使用SVM进行二分类并使用验证集,你可以使用sklearn库中的train_test_split函数将数据集划分为训练集和验证集。首先,你需要导入train_test_split函数。接下来,你可以按照以下步骤进行操作:
1. 将数据集拆分为特征和标签。特征是用于分类的数据,而标签是指示数据所属类别的值。
2. 使用train_test_split函数将数据集划分为训练集和验证集。该函数需要传入特征和标签两个参数,并设置test_size参数来指定验证集的比例。
3. 使用训练集数据拟合SVM模型,可以使用sklearn库中的SVM分类器。你可以根据需要选择合适的SVM模型,例如linearSVC或SVC。
4. 使用训练好的模型在验证集上进行预测,并计算准确率或其他评估指标来评估模型的性能。
下面是一个示例代码,展示了如何使用svm进行二分类并使用验证集来评估模型的性能:
```
# 导入所需的库和函数
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# 拆分数据集为特征和标签
features = your_features # 替换为你的特征数据
labels = your_labels # 替换为你的标签数据
# 划分数据集为训练集和验证集
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2)
# 创建SVM分类器模型
model = SVC()
# 在训练集上训练模型
model.fit(X_train, y_train)
# 在验证集上进行预测
y_pred = model.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
# 打印准确率
print("Accuracy: ", accuracy)
```
svm二分类python代码
当然!这里是一个基本的 SVM 二分类的 Python 代码示例:
```python
# 导入所需的库
from sklearn import svm
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 假设你已经有了训练数据 X 和对应的标签 y
# X 是一个二维数组,每一行代表一个样本的特征
# y 是一个一维数组,每个元素代表对应样本的标签
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建一个 SVM 模型
model = svm.SVC()
# 使用训练数据来训练模型
model.fit(X_train, y_train)
# 使用训练好的模型对测试集进行预测
y_pred = model.predict(X_test)
# 计算模型的准确率
accuracy = accuracy_score(y_test, y_pred)
# 打印准确率
print("Accuracy:", accuracy)
```
以上代码使用 `scikit-learn` 库中的 `SVC` 类来实现 SVM 模型的训练和预测。首先,我们将数据集分成训练集和测试集,然后创建一个 SVM 模型对象。接下来,使用训练数据来训练模型,并使用测试集来对模型进行评估,计算模型的准确率。
请注意,这只是一个基本的示例。在实际使用中,你可能需要根据你的数据集和问题进行一些调整和优化。
相关推荐
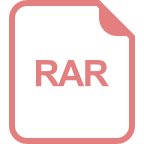
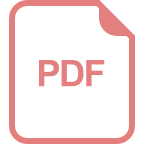












