给我一份jpeg压缩算法的代码
时间: 2023-07-10 10:03:19 浏览: 70
以下是一份简单的JPEG压缩算法C++代码:
```
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main(int argc, char **argv)
{
if(argc != 2)
{
cout << "Usage: ./jpeg_compress image.jpg" << endl;
return -1;
}
// 读取图像
Mat img = imread(argv[1], IMREAD_COLOR);
if(img.empty())
{
cout << "Failed to read image!" << endl;
return -1;
}
// 转换为YCrCb空间
Mat img_ycrcb;
cvtColor(img, img_ycrcb, COLOR_BGR2YCrCb);
// 分离通道
vector<Mat> channels;
split(img_ycrcb, channels);
// 对Y分量进行DCT变换
Mat y_dct;
dct(channels[0], y_dct);
// 量化
int q[8][8] = {{16, 11, 10, 16, 24, 40, 51, 61},
{12, 12, 14, 19, 26, 58, 60, 55},
{14, 13, 16, 24, 40, 57, 69, 56},
{14, 17, 22, 29, 51, 87, 80, 62},
{18, 22, 37, 56, 68, 109, 103, 77},
{24, 35, 55, 64, 81, 104, 113, 92},
{49, 64, 78, 87, 103, 121, 120, 101},
{72, 92, 95, 98, 112, 100, 103, 99}};
for(int i=0; i<y_dct.rows; i+=8)
{
for(int j=0; j<y_dct.cols; j+=8)
{
Mat block = y_dct(Rect(j, i, 8, 8));
block.convertTo(block, CV_32F);
for(int x=0; x<8; x++)
{
for(int y=0; y<8; y++)
{
block.at<float>(x, y) = round(block.at<float>(x, y) / q[x][y]);
}
}
block.convertTo(block, CV_16S);
}
}
// 反量化
for(int i=0; i<y_dct.rows; i+=8)
{
for(int j=0; j<y_dct.cols; j+=8)
{
Mat block = y_dct(Rect(j, i, 8, 8));
block.convertTo(block, CV_32F);
for(int x=0; x<8; x++)
{
for(int y=0; y<8; y++)
{
block.at<float>(x, y) = block.at<float>(x, y) * q[x][y];
}
}
block.convertTo(block, CV_16S);
}
}
// 对Y分量进行IDCT变换
Mat y_idct;
idct(y_dct, y_idct);
// 合并通道
channels[0] = y_idct;
Mat img_ycrcb_out;
merge(channels, img_ycrcb_out);
// 转换为BGR空间
Mat img_out;
cvtColor(img_ycrcb_out, img_out, COLOR_YCrCb2BGR);
// 保存图像
imwrite("compressed.jpg", img_out);
return 0;
}
```
该代码实现了以下步骤:
1. 读取图像并转换为YCrCb色彩空间
2. 分离通道
3. 对Y分量进行DCT变换
4. 量化
5. 反量化
6. 对Y分量进行IDCT变换
7. 合并通道并转换为BGR色彩空间
8. 保存压缩后的图像
这里使用了OpenCV库中的dct和idct函数,它们分别实现了离散余弦变换和离散余弦逆变换。值得注意的是,该代码中的量化表是固定的,而实际上JPEG压缩算法中采用的量化表是可调的,不同的量化表可以产生不同的压缩效果。
阅读全文
相关推荐
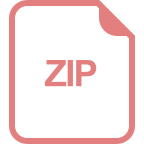
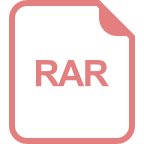
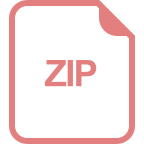
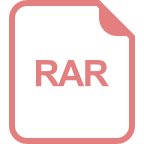
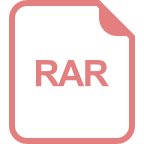
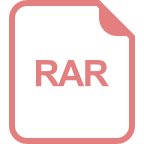
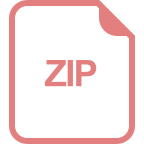
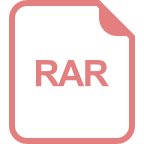
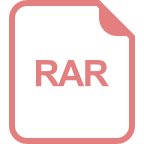
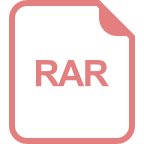
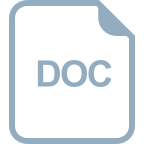
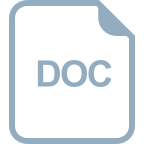
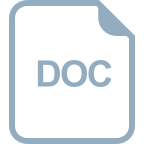
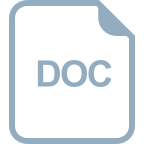
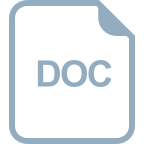
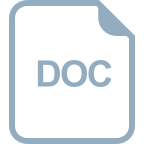