使用C#语言创建聊天机器人程序
时间: 2024-03-08 13:51:31 浏览: 205
好的,我可以为您提供一些关于如何使用 C# 语言创建聊天机器人程序的指导。
首先,您需要选择一个合适的机器人框架或库,比如 Microsoft Bot Framework,然后在 Visual Studio 中建立一个 C# 项目,并在项目中添加该框架或库。
接下来,您需要编写代码来处理用户的输入和输出。这可以通过使用框架或库中提供的 API 来实现,例如使用 LUIS(Language Understanding Intelligent Service)来理解用户的意图,然后使用相应的逻辑来回复用户。
您还需要创建一个适当的数据模型来存储机器人的知识库和响应数据。这可以使用数据库或其他类型的数据存储来完成。
最后,您还需要对聊天机器人程序进行测试和优化,以确保它能够正确地理解用户的输入并提供适当的响应。
以下是一个基本的示例代码,可以让您开始构建自己的聊天机器人程序:
```csharp
using Microsoft.Bot.Builder;
using Microsoft.Bot.Builder.Dialogs;
using Microsoft.Bot.Schema;
using System.Threading;
using System.Threading.Tasks;
namespace MyBot
{
public class MyBot : ActivityHandler
{
private readonly ConversationState _conversationState;
private readonly UserState _userState;
private readonly DialogSet _dialogs;
public MyBot(ConversationState conversationState, UserState userState)
{
_conversationState = conversationState;
_userState = userState;
// Create a new dialog set.
_dialogs = new DialogSet();
// Add a dialog that will greet the user and ask for their name.
_dialogs.Add(new WaterfallDialog("greeting", new WaterfallStep[]
{
async (stepContext, cancellationToken) =>
{
await stepContext.Context.SendActivityAsync("Hello! What's your name?");
return Dialog.EndOfTurn;
},
async (stepContext, cancellationToken) =>
{
string name = (string)stepContext.Result;
await stepContext.Context.SendActivityAsync($"Nice to meet you, {name}!");
return Dialog.EndOfTurn;
}
}));
// Add a dialog that will echo back any input from the user.
_dialogs.Add(new WaterfallDialog("echo", new WaterfallStep[]
{
async (stepContext, cancellationToken) =>
{
await stepContext.Context.SendActivityAsync("What would you like me to repeat?");
return Dialog.EndOfTurn;
},
async (stepContext, cancellationToken) =>
{
string message = (string)stepContext.Result;
await stepContext.Context.SendActivityAsync($"You said: {message}");
return Dialog.EndOfTurn;
}
}));
}
protected override async Task OnMessageActivityAsync(ITurnContext<IMessageActivity> turnContext, CancellationToken cancellationToken)
{
var dc = await _dialogs.CreateContextAsync(turnContext, cancellationToken);
// Run the appropriate dialog based on the user's input.
if (turnContext.Activity.Text.ToLower().Contains("hello"))
{
await dc.BeginDialogAsync("greeting", null, cancellationToken);
}
else
{
await dc.BeginDialogAsync("echo", null, cancellationToken);
}
}
}
}
```
希望这些指导对您有所帮助。如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
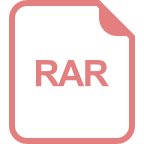
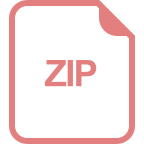
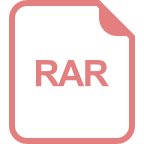
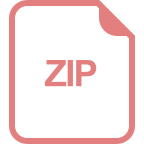
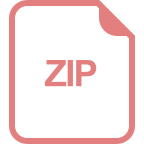
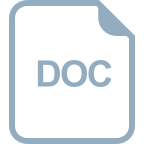


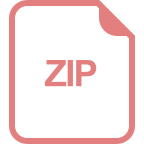
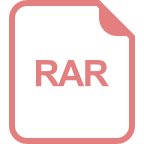
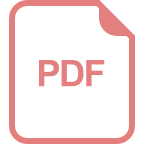
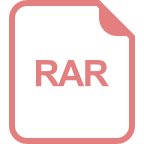
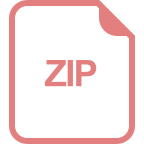
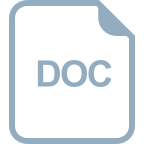
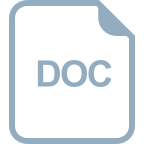
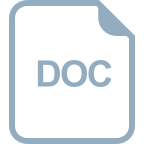