我用C语言通过ShellExecute函数打开word文档时,希望判断出执行的环境是否安装能正常打开word文档的office软件,有代码能够实现吗
时间: 2024-03-05 19:51:24 浏览: 18
可以使用Registry API来检查注册表中是否存在Office的安装信息,从而判断是否安装了Office软件。以下是一个示例代码:
```c
#include <windows.h>
#include <stdio.h>
#include <tchar.h>
int CheckOfficeInstalled()
{
HKEY hKey;
TCHAR szOfficePath[MAX_PATH];
DWORD cbData = sizeof(szOfficePath);
LONG lResult;
// 检查 Office 2019
lResult = RegOpenKeyEx(HKEY_CLASSES_ROOT, _T("Word.Application\\CurVer"), 0, KEY_QUERY_VALUE, &hKey);
if (lResult == ERROR_SUCCESS)
{
lResult = RegQueryValueEx(hKey, _T(""), NULL, NULL, (LPBYTE)szOfficePath, &cbData);
if (lResult == ERROR_SUCCESS)
{
if (_tcsstr(szOfficePath, _T("Office16")) != NULL)
{
_tprintf(_T("Office 2019 installed\n"));
return TRUE;
}
}
RegCloseKey(hKey);
}
// 检查 Office 2016
lResult = RegOpenKeyEx(HKEY_CLASSES_ROOT, _T("Word.Application.16\\CurVer"), 0, KEY_QUERY_VALUE, &hKey);
if (lResult == ERROR_SUCCESS)
{
lResult = RegQueryValueEx(hKey, _T(""), NULL, NULL, (LPBYTE)szOfficePath, &cbData);
if (lResult == ERROR_SUCCESS)
{
if (_tcsstr(szOfficePath, _T("Office16")) != NULL)
{
_tprintf(_T("Office 2016 installed\n"));
return TRUE;
}
}
RegCloseKey(hKey);
}
// 检查 Office 2013
lResult = RegOpenKeyEx(HKEY_CLASSES_ROOT, _T("Word.Application.15\\CurVer"), 0, KEY_QUERY_VALUE, &hKey);
if (lResult == ERROR_SUCCESS)
{
lResult = RegQueryValueEx(hKey, _T(""), NULL, NULL, (LPBYTE)szOfficePath, &cbData);
if (lResult == ERROR_SUCCESS)
{
if (_tcsstr(szOfficePath, _T("Office15")) != NULL)
{
_tprintf(_T("Office 2013 installed\n"));
return TRUE;
}
}
RegCloseKey(hKey);
}
// 检查 Office 2010
lResult = RegOpenKeyEx(HKEY_CLASSES_ROOT, _T("Word.Application.14\\CurVer"), 0, KEY_QUERY_VALUE, &hKey);
if (lResult == ERROR_SUCCESS)
{
lResult = RegQueryValueEx(hKey, _T(""), NULL, NULL, (LPBYTE)szOfficePath, &cbData);
if (lResult == ERROR_SUCCESS)
{
if (_tcsstr(szOfficePath, _T("Office14")) != NULL)
{
_tprintf(_T("Office 2010 installed\n"));
return TRUE;
}
}
RegCloseKey(hKey);
}
// 没有检测到 Office
_tprintf(_T("Office not installed\n"));
return FALSE;
}
int main()
{
CheckOfficeInstalled();
return 0;
}
```
该代码检查 Office 2010 到 Office 2019 的安装信息,并输出检测到的 Office 版本。如果没有检测到 Office,则输出 "Office not installed"。你可以在打开 Word 文档前,调用 CheckOfficeInstalled() 函数来判断是否安装了 Office 软件。
相关推荐
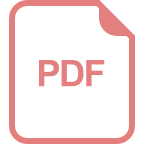
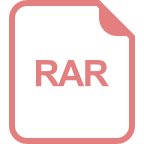














