用C语言实现输出公元1900年至公元2500年所有闰年的年份,没输出5个年份换一行(闰年的判断条件是,年份能被4整除,而不能被100整除,或能被400整除)
时间: 2023-08-31 17:20:27 浏览: 269
### 回答1:
以下是用C语言实现输出公元1900年至公元2500年所有闰年的年份的代码:
```c
#include <stdio.h>
int main() {
int year, count = 0;
for (year = 1900; year <= 2500; year++) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
printf("%d ", year);
count++;
if (count % 5 == 0) {
printf("\n");
}
}
}
return 0;
}
```
代码中使用 for 循环遍历从公元1900年至公元2500年的所有年份,使用 if 语句判断是否为闰年,如果是则输出该年份,并且计数器 count 加 1。当 count 的值是 5 的倍数时,使用 printf 函数输出换行符,以实现每输出5个年份换一行的要求。最后返回0表示程序运行结束。
### 回答2:
以下是用C语言实现输出公元1900年至公元2500年所有闰年的年份的代码:
```c
#include <stdio.h>
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return 1; // 是闰年
} else {
return 0; // 不是闰年
}
}
int main() {
int count = 0;
for (int year = 1900; year <= 2500; year++) {
if (isLeapYear(year)) {
printf("%d ", year);
count++;
}
if (count % 5 == 0 && count != 0) {
printf("\n");
}
}
return 0;
}
```
上述代码中,首先定义了一个`isLeapYear`函数,该函数判断给定的年份`year`是否为闰年,其条件为能被4整除且不能被100整除,或者能被400整除。
在`main`函数中,使用一个循环从1900年到2500年遍历每一个年份,并通过调用`isLeapYear`函数判断是否为闰年。如果是闰年,则输出年份,并将计数器`count`递增。当`count`的值能被5整除且不为0时,说明已经输出了5个年份,需要换行。
运行以上代码,就可以得到输出公元1900年至公元2500年所有闰年的年份。
阅读全文
相关推荐
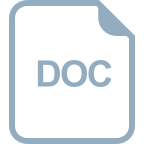














