linux aio使用示例
时间: 2024-05-14 12:10:29 浏览: 9
Linux AIO(Asynchronous I/O)是一种异步I/O机制,它可以让应用程序在进行I/O操作时,不必等待I/O操作完成才返回,而是在I/O操作进行的同时可以继续执行其他任务。这种机制可以极大地提高I/O操作的效率和应用程序的并发性能。
下面是一个简单的Linux AIO使用示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <aio.h>
#include <errno.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
int fd, ret;
char buf[BUFSIZE];
struct aiocb aiocb;
// 打开文件
fd = open(argv, O_RDONLY);
if (fd < 0) {
perror("open");
exit(1);
}
// 初始化aiocb结构体
bzero(&aiocb, sizeof(struct aiocb));
aiocb.aio_fildes = fd;
aiocb.aio_buf = buf;
aiocb.aio_nbytes = BUFSIZE;
aiocb.aio_offset = 0;
// 异步读取文件
ret = aio_read(&aiocb);
if (ret < 0) {
perror("aio_read");
exit(1);
}
// 等待异步读取完成
while (aio_error(&aiocb) == EINPROGRESS) {
printf("reading...\n");
sleep(1);
}
// 检查异步读取结果
ret = aio_return(&aiocb);
if (ret < 0) {
perror("aio_return");
exit(1);
}
// 输出读取结果
printf("read %d bytes: %s\n", ret, buf);
// 关闭文件
close(fd);
return 0;
}
```
以上代码会打开指定的文件,异步读取文件内容,等待异步读取完成,输出读取结果,并关闭文件。在这个示例中,aio_read()函数会立即返回,不会阻塞程序运行。如果要进行多个异步I/O操作,可以使用aio_suspend()函数等待所有异步I/O操作完成。
相关推荐
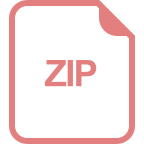
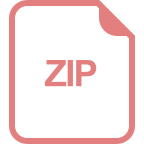














