vue2自动识别图片内两直线交点坐标完整代码
时间: 2024-12-12 07:22:29 浏览: 11
Vue.js本身是一个前端框架,用于构建用户界面,并非图像处理库,因此它不会直接提供图片分析功能来计算两条直线的交点坐标。然而,你可以结合一些前端图像处理库,比如`html2canvas`或`fabric.js`,配合JavaScript的数学库如`math.js`来实现这个需求。
下面是一个简化的示例,使用`fabric.js`处理图片并计算两点连线的交点。首先安装必要的依赖:
```bash
npm install fabric.js mathjs
```
然后,在Vue组件中使用它们:
```html
<template>
<div ref="imageCanvas" style="width: 400px; height: 400px;"></div>
</template>
<script>
import * as fabric from 'fabric';
import { pointLineIntersection } from 'mathjs';
export default {
data() {
return {
canvas: null,
line1: { x1: 50, y1: 50, x2: 350, y2: 250 },
line2: { x1: 150, y1: 150, x2: 300, y2: 300 },
};
},
mounted() {
this.initFabric();
},
methods: {
initFabric() {
this.canvas = new fabric.Canvas('imageCanvas');
const imgElement = document.createElement('img');
imgElement.src = 'your_image_url'; // 替换为你需要处理的图片URL
imgElement.onload = () => {
this.canvas.setWidth(imgElement.width);
this.canvas.setHeight(imgElement.height);
this.canvas.loadFromURL(imgElement.src, (canvas, img) => {
canvas.renderAll();
// 添加线条到画布
const line1 = new fabric.Line(this.line1, { selectable: false });
const line2 = new fabric.Line(this.line2, { selectable: false });
// 计算交点
const intersection = pointLineIntersection(line1.getXY(), line2.getXY());
console.log('Intersection:', intersection); // 打印结果
// 如果有需求,可以在canvas上添加标记交点
if (intersection) {
const intersectionMarker = new fabric.Circle({
left: intersection[0],
top: intersection[1],
radius: 5,
fill: 'red',
});
this.canvas.add(intersectionMarker);
}
});
};
},
},
};
</script>
```
注意,这个例子假设图片中的直线是水平或垂直的,对于一般斜线,你需要更复杂的算法来计算交点。此外,这只是一个基本示例,实际应用中可能需要考虑性能优化,特别是处理大图时。
阅读全文
相关推荐
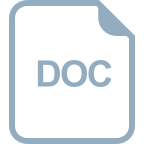
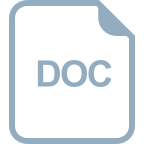
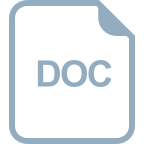
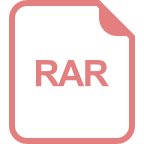
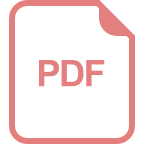
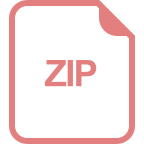
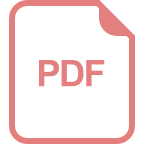
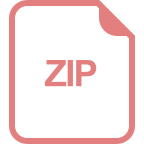
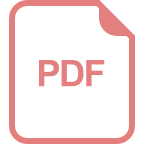
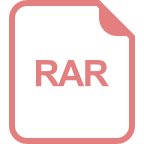
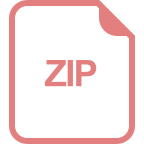
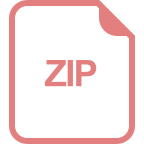
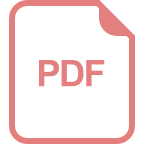
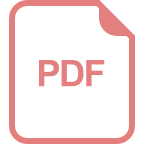