用c语言表示数据结构线性表操作和算法代码
时间: 2024-09-19 11:07:37 浏览: 49
在C语言中,我们可以使用数组或链表这两种基本的数据结构来表示线性表,并进行各种操作。这里我会给出一些常见的线性表操作及相应的代码示例。
1. **数组表示** (固定大小):
- 创建空数组:`int list[5];`
- 插入/删除元素:由于数组大小固定,插入或删除通常需要移动其他元素。例如,插入元素到末尾:
```c
void insert_array(int *list, int value, size_t n) {
if (n < sizeof(list) / sizeof(*list)) {
list[n] = value;
} else {
printf("Array full.\n");
}
}
```
- 访问元素:`printf("%d ", list[i]);`
2. **动态链表表示** (动态大小):
- 定义节点结构体:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
- 创建链表:
```c
Node* create_list() {
Node* head = NULL;
return head;
}
```
- 插入元素:
```c
void insert_node(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
- 删除元素:
```c
void delete_node(Node** head, int value) {
Node* current = *head, *prev = NULL;
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
if (current == NULL) return; // not found
if (prev == NULL) *head = current->next;
else prev->next = current->next;
free(current);
}
```
阅读全文
相关推荐
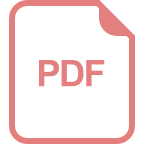
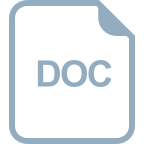
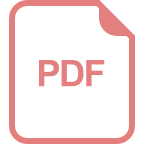
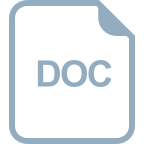
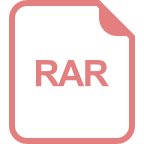
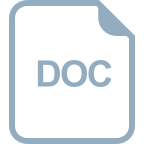
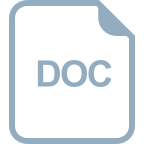


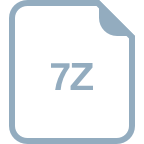
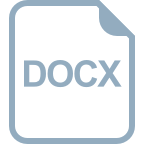
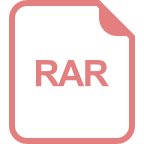
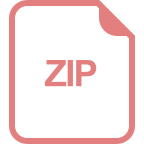
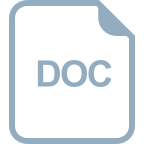
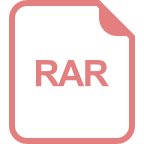
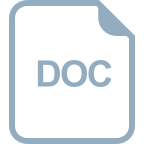
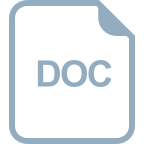
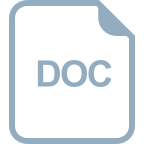
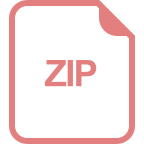