s = batch['A'].cuda() TypeError: list indices must be integers or slices, not str
时间: 2024-08-14 08:06:42 浏览: 42
这个错误提示是在PyTorch编程中遇到的问题。当你尝试通过索引访问一个名为`batch`的字典,并期望获取键为'A'的值,但是`'A'`实际上是一个字符串而不是整数或切片。在PyTorch的`torch.utils.data.DataLoader`返回的数据结构中,通常使用整数下标去访问批量数据,而不会直接使用字符串。
正确的做法应该是先检查`'A'`是否在`batch`的键中,然后用对应的整数值去访问,例如:
```python
if 'A' in batch:
a_batch = batch['A']
else:
# 或者处理键不存在的情况
raise KeyError("Key 'A' not found in the batch")
a_batch = a_batch.cuda() # 如果A是一个可以转换到GPU的类型
```
相关问题
TypeError: list indices must be integers or slices, not tuple 给出解决代码
这个错误通常出现在试图使用tuple作为list的索引时。在这里,可能是在使用batch_idx变量时出现了这个错误。你可以尝试把batch_idx改为batch_idx来解决这个问题。下面是可能的解决代码:
```python
# test the model
with torch.no_grad():
correct = 0
total = 0
for batch_idx, batch_data in enumerate(test_dataloader):
x = batch_data
y = batch_data
outputs = model(x)
predicted = torch.argmax(outputs, dim=1)
total += y.size(0)
correct += (predicted == y).sum().item()
print('Accuracy of the model on the test data: {} %'.format(100 * correct / total))
```
for epoch in range(100): for batch in dataloader: optimizer.zero_grad() x = batch[:, :-1, :] y = batch[:, 1:, :]报错TypeError: list indices must be integers or slices, not tuple
这个错误很可能是因为你的dataloader返回的是元组而不是列表。你可以尝试将其转换为列表,如下所示:
```
for epoch in range(100):
for batch in dataloader:
optimizer.zero_grad()
batch = list(batch)
x = batch[:, :-1, :]
y = batch[:, 1:, :]
```
或者你可以在创建dataloader时指定返回的元素类型为列表,如下所示:
```
dataloader = DataLoader(dataset, batch_size=32, collate_fn=list)
```
阅读全文
相关推荐
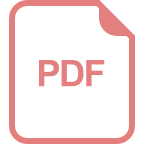
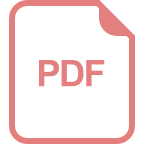
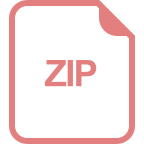
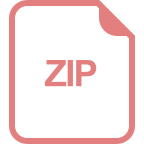
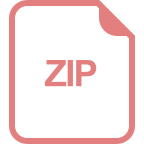
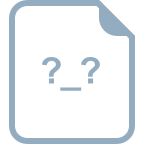
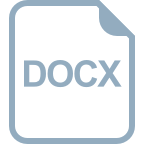
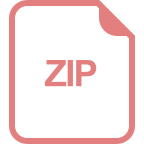
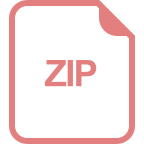
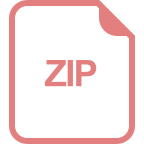
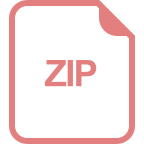
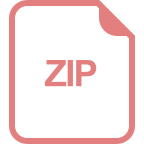
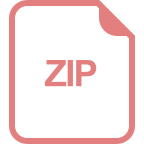