在java中定义一个有3个元素的整形数组a,并赋值。循环遍历下标从0-4 (不含), 输出a的元素,并求平均值。捕捉下标越界异常,除数为0异常,其他异常。
时间: 2024-11-15 22:22:28 浏览: 15
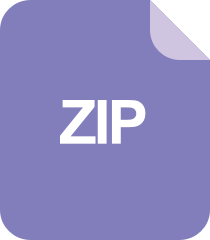
Java数组定义与遍历练习题
在Java中,你可以使用`ArrayIndexOutOfBoundsException`异常来处理索引超出数组范围的情况,而`ArithmeticException`则用于捕获除以零的异常。下面是创建一个3元素的整型数组并进行遍历、输出元素以及计算平均值的示例代码:
```java
public class Main {
public static void main(String[] args) {
int[] a = new int[3]; // 创建一个长度为3的整型数组
try {
// 初始化数组元素(这里假设我们只初始化前三个元素)
a[0] = 5;
a[1] = 10;
a[2] = 15;
// 循环遍历下标0到2(因为数组索引是从0开始的,所以最大到2不包含4)
for (int i = 0; i <= 2; i++) {
System.out.println("Element at index " + i + ": " + a[i]);
}
// 计算平均值(这里没有第四个元素,所以不需要除以4)
double average = calculateAverage(a);
System.out.println("Average value: " + average);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Error: Index out of bounds. Make sure you're not accessing an array element beyond its length.");
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero is not allowed.");
} catch (Exception e) { // 捕获所有其他未知异常
System.out.println("An unexpected error occurred: " + e.getMessage());
}
}
// 方法来计算数组元素的平均值
private static double calculateAverage(int[] numbers) {
if (numbers.length == 0) {
throw new ArithmeticException("Cannot calculate average with an empty array");
}
return sum(numbers) / numbers.length;
}
// 辅助方法来计算数组元素总和
private static int sum(int[] arr) {
int total = 0;
for (int num : arr) {
total += num;
}
return total;
}
}
```
在这个代码中,如果你尝试访问索引`i > 2`的元素,将会抛出`ArrayIndexOutOfBoundsException`;如果在计算平均值时执行了除以零的操作(例如数组为空),将抛出`ArithmeticException`。
阅读全文
相关推荐
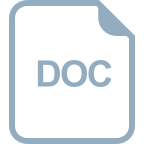
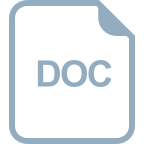
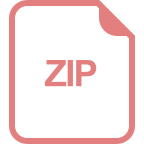
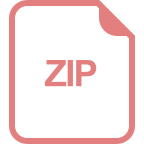
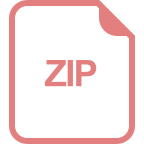
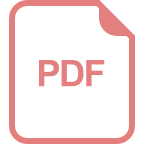
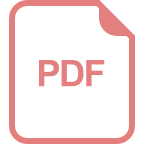
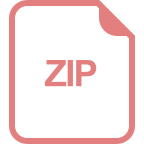
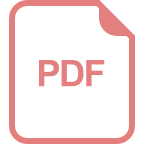
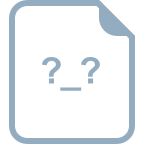
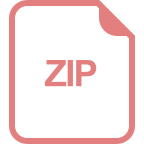
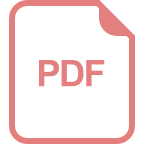
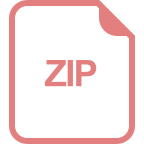
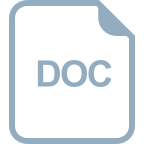
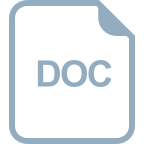
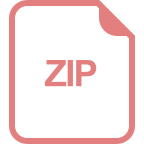
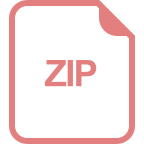