c#中虚拟方法和抽象方法的异同
时间: 2024-06-13 14:09:42 浏览: 8
在C#中,虚拟方法和抽象方法都是面向对象编程中的重要概念,它们的异同点如下:
相同点:
- 都是用来实现多态性的机制。
- 都不能被实例化。
不同点:
- 抽象方法必须在抽象类中声明,而虚拟方法则不需要。
- 抽象方法没有实现,而虚拟方法有默认实现。
- 子类必须实现抽象方法,而虚拟方法可以选择性地覆盖。
- 抽象方法没有方法体,而虚拟方法有方法体。
- 抽象方法必须使用abstract关键字声明,而虚拟方法必须使用virtual关键字声明。
下面是一个简单的示例,演示了如何在C#中使用抽象方法和虚拟方法:
```csharp
// 抽象类
abstract class Shape
{
public abstract void Draw(); // 抽象方法
}
// 派生类
class Circle : Shape
{
public override void Draw() // 实现抽象方法
{
Console.WriteLine("Drawing a circle");
}
public virtual void Erase() // 虚拟方法
{
Console.WriteLine("Erasing a circle");
}
}
// 另一个派生类
class Square : Shape
{
public override void Draw() // 实现抽象方法
{
Console.WriteLine("Drawing a square");
}
}
// 测试代码
static void Main(string[] args)
{
Shape[] shapes = new Shape[2];
shapes[0] = new Circle();
shapes[1] = new Square();
foreach (Shape shape in shapes)
{
shape.Draw(); // 调用抽象方法
}
Circle circle = new Circle();
circle.Erase(); // 调用虚拟方法
Console.ReadLine();
}
```
相关推荐
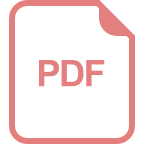
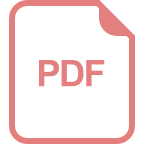
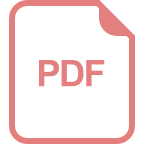
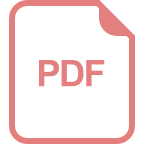
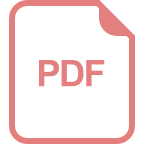
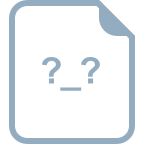
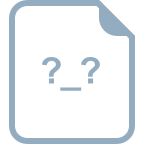
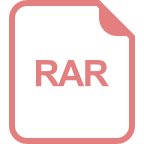