c++文件流file
时间: 2024-12-30 15:30:12 浏览: 9
### C++ 文件流操作
#### 使用 `ofstream` 进行文件写入
为了向文件中写入数据,可以使用 `ofstream` 类。此对象允许程序创建新文件或将现有文件截断为零长度并准备写入[^1]。
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outfile;
outfile.open("example.txt");
if (!outfile.is_open()) {
cerr << "Unable to open file";
return 1;
}
outfile << "Writing this line into the file." << endl;
outfile.close();
}
```
这段代码展示了如何打开名为 `"example.txt"` 的文件,并向其中写入一条消息。如果文件不存在,则会自动创建;如果存在则会被清空以便重新写入[^3]。
#### 使用 `ifstream` 实现文件读取
对于从文件读取内容的任务,应该利用 `ifstream` 对象。这使得能够逐字符或按行读取文件的内容[^2]。
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream infile("example.txt");
string str;
while (getline(infile, str)) {
cout << str << endl;
}
infile.close();
}
```
上述例子说明了怎样通过循环遍历每一行直到遇到文件结束符 EOF 来完成整个文件的读取过程。
#### 获取二进制文件大小
当处理二进制文件时,可能需要知道其确切尺寸。下面的例子演示了计算给定路径下指定名称的二进制文件的实际字节数量的方法[^4]:
```cpp
#include <iostream>
#include <fstream>
const char* filename = "test.bin";
int main() {
ifstream in(filename, ios::in | ios::binary);
if (!in) {
cerr << "Cannot open file\n";
return 1;
}
// Move pointer to end and get position which equals size.
in.seekg(0, ios::end);
auto fileSize = in.tellg();
cout << "Size of " << filename << ": " << fileSize << " bytes.\n";
in.close();
}
```
这里的关键在于调用了成员函数 `seekg()` 将内部位置指针移动至文件结尾处 (`ios::end`) 并随后获取当前位置即代表该文件总字节长度。
阅读全文
相关推荐










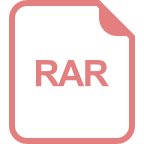






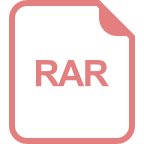