c++写一个倒计时组件
时间: 2024-09-11 19:10:36 浏览: 50
在C++中创建一个倒计时组件,可以通过多种方式实现,其中一种常见的方法是使用线程或者定时器来处理时间的减少和事件的触发。下面是一个简单的例子,使用标准库中的`<chrono>`和`<thread>`来实现一个简单的倒计时功能。
```cpp
#include <iostream>
#include <chrono>
#include <thread>
class Countdown {
public:
explicit Countdown(int duration) : duration_(duration) {}
void Start() {
using namespace std::chrono;
while (duration_ > 0) {
// 获取当前时间点
steady_clock::time_point now = steady_clock::now();
// 计算剩余时间
steady_clock::duration remaining = steady_clock::duration::max() - now.time_since_epoch();
auto sleep_time = std::min(remaining, std::chrono::seconds(duration_));
// 暂停执行一段时间
std::this_thread::sleep_for(sleep_time);
// 倒计时减少
duration_ -= static_cast<int>(sleep_time.count());
std::cout << "剩余时间: " << duration_ << " 秒" << std::endl;
}
std::cout << "倒计时结束!" << std::endl;
}
private:
int duration_; // 倒计时总时间,单位为秒
};
int main() {
int total_seconds;
std::cout << "请输入倒计时时间(秒): ";
std::cin >> total_seconds;
if (total_seconds <= 0) {
std::cout << "请输入一个大于0的整数。" << std::endl;
return 1;
}
Countdown countdown(total_seconds);
countdown.Start();
return 0;
}
```
这个例子中的`Countdown`类接受一个整数参数表示倒计时的总时间,`Start`方法中使用了`std::this_thread::sleep_for`来让当前线程暂停指定的时间,每经过一秒,倒计时的时间就减少一秒,直到时间耗尽。
注意:这个简单的例子并没有处理跨秒的时间差,如果需要精确到每个整秒,可以调整`std::this_thread::sleep_for`中的`sleep_time`计算方式。
阅读全文
相关推荐
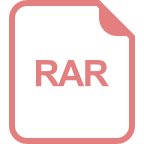
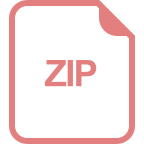
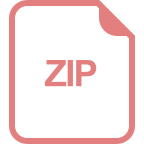
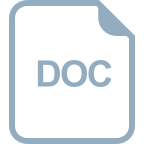

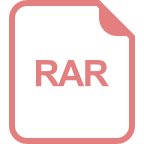
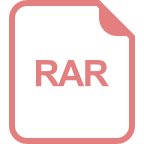
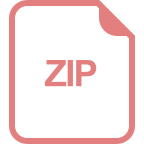
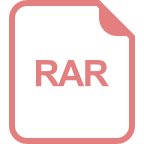
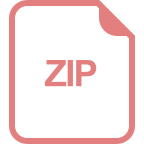
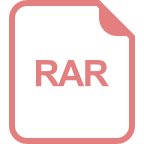
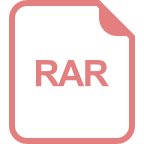
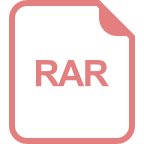
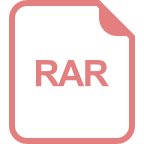
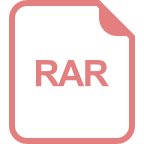
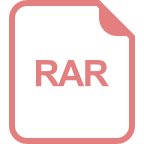
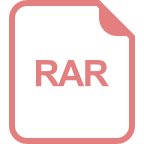
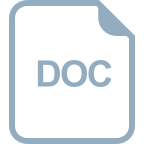
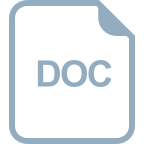