使用vs2024编译,假设串采用顺序串储存,设计一个算法Strcmp(s,t)按字典顺序比较两个串s和t的大小
时间: 2024-12-12 15:26:32 浏览: 7
在使用 Visual Studio 2024 编译环境中,如果字符串 `s` 和 `t` 都是顺序存储的(例如字符数组或 C++ 的 std::string 类型),你可以设计一个简单的逐字符比较的算法来进行strcmp操作。这里有一个基本的C++实现示例:
```cpp
#include <string>
// 比较两个字符串是否相等,若相等则返回0,s小于t返回负数,s大于t返回正数
int Strcmp(const char* s, const char* t) {
while (*s != '\0' && *t != '\0') { // 当两者都未到达字符串结束符
if (*s < *t) return -1; // 如果当前字符s小于t,则s更小
else if (*s > *t) return 1; // 如果当前字符s大于t,则s更大
s++; t++; // 否则,继续比较下一个字符
}
// 如果其中一个字符串先到达结尾,另一个还没到,则剩下的部分较长的那个更大
if (*s == '\0' && *t != '\0') return 1;
else if (*s != '\0' && *t == '\0') return -1;
// 若两个字符串长度相等且所有字符都相等,则它们相等
return 0;
}
```
相关问题
用C语言实现一个函数功能 输入:一个json文件, 要求:在这个json文件中增加一个参数“sig”,参数的值sign需要根据json文件内容计算得出。输出新的json文件。 参数的计算方法: 第一步: 将json文件所有非空参数拼接成一个stringA,拼接规则: 按照参数名ASCII码从小到大排序(字典序),使用URL键值对的格式(即key1=value1&key2=value2…)拼接成字符串 第二步:对stringA使用key进行HmacSHA256算法签名,得到sign
以下是一个使用C语言实现的函数,可以实现上述要求:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <openssl/hmac.h>
#include "cJSON.h"
char* calculate_sign(char* json_file, char* key) {
cJSON* root = cJSON_Parse(json_file);
if (!root) {
printf("Error parsing json file!\n");
return NULL;
}
int size = cJSON_GetArraySize(root);
char** keys = (char**) malloc(size * sizeof(char*));
char** values = (char**) malloc(size * sizeof(char*));
int i = 0;
cJSON* child = root->child;
while (child) {
char* key = child->string;
char* value = cJSON_PrintUnformatted(child);
keys[i] = key;
values[i] = value;
child = child->next;
i++;
}
// Sort keys in ascending order (using bubble sort)
for (i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (strcmp(keys[j], keys[j+1]) > 0) {
char* temp = keys[j];
keys[j] = keys[j+1];
keys[j+1] = temp;
temp = values[j];
values[j] = values[j+1];
values[j+1] = temp;
}
}
}
// Concatenate keys and values into a string
char* stringA = (char*) malloc(4096);
stringA[0] = '\0';
for (i = 0; i < size; i++) {
strcat(stringA, keys[i]);
strcat(stringA, "=");
strcat(stringA, values[i]);
if (i < size - 1) {
strcat(stringA, "&");
}
}
// Calculate HMAC-SHA256 of stringA using key
unsigned int len = strlen(key);
unsigned char* hmac = HMAC(EVP_sha256(), key, len, (unsigned char*)stringA, strlen(stringA), NULL, NULL);
// Convert HMAC to hexadecimal string
char* sign = (char*) malloc(65);
for (i = 0; i < 32; i++) {
sprintf(&sign[i*2], "%02x", hmac[i]);
}
sign[64] = '\0';
// Add "sig" parameter to the JSON object
cJSON_AddStringToObject(root, "sig", sign);
// Convert the JSON object back into a string
char* new_json_file = cJSON_PrintUnformatted(root);
// Free memory
for (i = 0; i < size; i++) {
free(keys[i]);
free(values[i]);
}
free(keys);
free(values);
free(stringA);
free(sign);
cJSON_Delete(root);
return new_json_file;
}
```
使用示例:
```c
int main() {
char* json_file = "{\"foo\": 123, \"bar\": \"hello\"}";
char* key = "my_secret_key";
char* new_json_file = calculate_sign(json_file, key);
printf("%s\n", new_json_file);
free(new_json_file);
return 0;
}
```
注意:需要在编译时链接 OpenSSL 库。例如,在 Linux 上可以使用以下命令编译:
```
gcc -o main main.c -lcrypto
```
LZW压缩算法及应用 基本要求 ① 在一个文本文件上实现LZW压缩和解压缩,其中每个字符就是该文本的8位ASCII码。 ② 在实现LZW过程中需要仔细考虑如何在编译表中找到匹配或找不到匹配,需要注意匹配算法的时间、空间开销。用c语言编写一个完整的代码可运行的
LZW压缩算法是一种常用的无损压缩算法,它可以在不损失数据的情况下减小文件的大小。以下是一个基于C语言的LZW压缩和解压缩程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DICT_SIZE 4096 // 最大字典大小
#define MAX_CODE_LEN 12 // 最大编码长度
unsigned char buffer[MAX_DICT_SIZE]; // 字典缓冲区
int dict[MAX_DICT_SIZE]; // 字典表
int dict_size; // 字典大小
// 初始化字典
void init_dict() {
for (int i = 0; i < 256; i++) {
buffer[i] = i;
dict[i] = i;
}
dict_size = 256;
}
// 压缩函数
void compress(FILE* input, FILE* output) {
int code = 0;
int next_code = 256;
int len = 0;
int c = fgetc(input);
while (c != EOF) {
buffer[len++] = c;
while (len > 0) {
int i = 0;
while (i < len && dict[code + buffer[i]] != 0) {
code += dict[code + buffer[i]];
i++;
}
if (i == len) {
break;
}
fputc(code, output);
dict[code + buffer[i]] = next_code++;
code = buffer[i];
len -= i;
memmove(buffer, buffer + i, len);
if (next_code >= MAX_DICT_SIZE) {
init_dict();
next_code = 256;
}
}
c = fgetc(input);
}
if (len > 0) {
fputc(code, output);
}
}
// 解压缩函数
void decompress(FILE* input, FILE* output) {
int code = 0;
int next_code = 256;
int len = 0;
int c = fgetc(input);
while (c != EOF) {
buffer[len++] = c;
if (len >= MAX_CODE_LEN) {
fprintf(stderr, "Error: Maximum code length exceeded.");
exit(1);
}
if (dict[c] != 0) {
code = dict[c];
} else if (c == next_code) {
code = buffer[0];
} else {
fprintf(stderr, "Error: Invalid compressed data.");
exit(1);
}
fputc(code, output);
dict[next_code++] = dict[c] + buffer[0];
if (next_code >= MAX_DICT_SIZE) {
init_dict();
next_code = 256;
}
c = fgetc(input);
}
}
int main(int argc, char* argv[]) {
if (argc != 4) {
fprintf(stderr, "Usage: lzw <mode> <input file> <output file>\n");
exit(1);
}
char* mode = argv[1];
char* input_file = argv[2];
char* output_file = argv[3];
FILE* input = fopen(input_file, "rb");
FILE* output = fopen(output_file, "wb");
if (input == NULL || output == NULL) {
fprintf(stderr, "Error: Failed to open file.\n");
exit(1);
}
init_dict();
if (strcmp(mode, "-c") == 0) {
compress(input, output);
} else if (strcmp(mode, "-d") == 0) {
decompress(input, output);
} else {
fprintf(stderr, "Error: Invalid mode.\n");
exit(1);
}
fclose(input);
fclose(output);
return 0;
}
```
上述代码中,`compress()`函数实现了LZW压缩算法,`decompress()`函数实现了LZW解压缩算法。`init_dict()`函数用于初始化字典表。在压缩和解压缩过程中,字典表的大小最多可以达到4096个条目,每个条目由12位编码组成。如果字典表大小达到了4096个条目,则程序将重新初始化字典表并从256重新开始编码。
在程序中,`compress()`函数将读入的数据与字典表中的编码进行匹配,并将匹配的编码写入输出文件。如果找不到匹配的编码,则将当前匹配的字符串添加到字典表中,并将其编码写入输出文件。`decompress()`函数则直接读取输入文件中的编码,并在字典表中查找对应的字符串。
注意:由于LZW算法产生的编码可能会超过8位,因此在压缩和解压缩过程中需要使用16位或更高位的整数来存储编码。在本例中,我们使用了32位整数来存储编码,但实际上只用到了12位。另外,本例中使用了文件输入输出,实际应用中可能需要进行适当的修改。
阅读全文
相关推荐
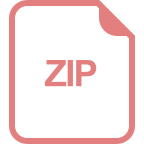
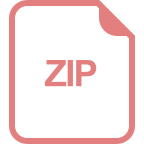
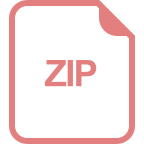
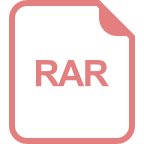
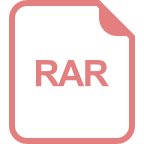
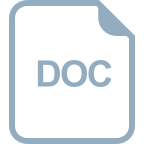
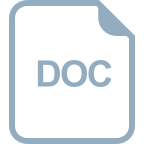
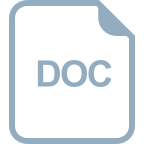
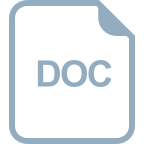
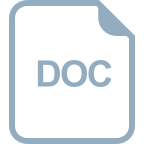
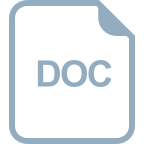
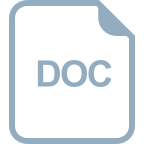
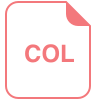
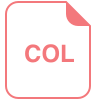
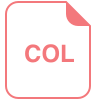
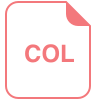
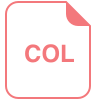