c语言 读取windows系统分区表
时间: 2023-08-24 14:36:45 浏览: 120
要在C语言中读取Windows系统分区表,可以使用Windows API函数来实现。以下是使用Windows API函数读取系统分区表的示例代码:
```c
#include <stdio.h>
#include <windows.h>
#include <winioctl.h>
int main()
{
HANDLE hDevice = CreateFile("\\\\.\\PhysicalDrive0", GENERIC_READ, FILE_SHARE_READ | FILE_SHARE_WRITE, NULL, OPEN_EXISTING, 0, NULL);
if (hDevice == INVALID_HANDLE_VALUE)
{
printf("Failed to open disk! Error code: %d\n", GetLastError());
return 1;
}
STORAGE_PROPERTY_QUERY storagePropertyQuery;
ZeroMemory(&storagePropertyQuery, sizeof(storagePropertyQuery));
storagePropertyQuery.PropertyId = StorageDeviceProperty;
storagePropertyQuery.QueryType = PropertyStandardQuery;
STORAGE_DEVICE_DESCRIPTOR storageDeviceDescriptor;
ZeroMemory(&storageDeviceDescriptor, sizeof(storageDeviceDescriptor));
DWORD dwBytesReturned;
if (!DeviceIoControl(hDevice, IOCTL_STORAGE_QUERY_PROPERTY, &storagePropertyQuery, sizeof(storagePropertyQuery), &storageDeviceDescriptor, sizeof(storageDeviceDescriptor), &dwBytesReturned, NULL))
{
printf("Failed to get device descriptor! Error code: %d\n", GetLastError());
CloseHandle(hDevice);
return 1;
}
BYTE* buffer = (BYTE*)malloc(storageDeviceDescriptor.Size);
ZeroMemory(buffer, storageDeviceDescriptor.Size);
if (!DeviceIoControl(hDevice, IOCTL_DISK_GET_DRIVE_LAYOUT_EX, NULL, 0, buffer, storageDeviceDescriptor.Size, &dwBytesReturned, NULL))
{
printf("Failed to get partition table! Error code: %d\n", GetLastError());
CloseHandle(hDevice);
free(buffer);
return 1;
}
PDRIVE_LAYOUT_INFORMATION_EX driveLayoutInformation = (PDRIVE_LAYOUT_INFORMATION_EX)buffer;
printf("Drive layout:\n");
printf("Signature: 0x%08X\n", driveLayoutInformation->Mbr.Signature);
for (int i = 0; i < driveLayoutInformation->PartitionCount; i++)
{
PARTITION_INFORMATION_EX partitionInformation = driveLayoutInformation->PartitionEntry[i].PartitionInformation;
printf("\nPartition %d:\n", i + 1);
printf("Partition type: 0x%02X\n", partitionInformation.PartitionType);
printf("Partition number: %d\n", partitionInformation.PartitionNumber);
printf("Starting offset: %lld\n", partitionInformation.StartingOffset.QuadPart);
printf("Partition length: %lld\n", partitionInformation.PartitionLength.QuadPart);
}
CloseHandle(hDevice);
free(buffer);
return 0;
}
```
这段代码打开物理磁盘设备,并使用DeviceIoControl函数发送IOCTL_DISK_GET_DRIVE_LAYOUT_EX控制码来获取分区表数据。然后,它解析分区表数据并将其打印到控制台上。请注意,这需要管理员权限才能运行。
阅读全文
相关推荐
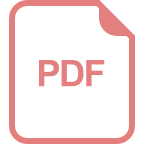
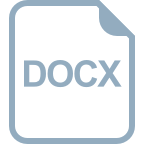
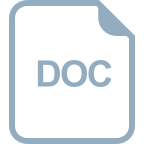















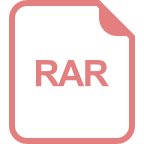