C++实现SHA-1算法
时间: 2023-12-01 18:56:07 浏览: 146
以下是C++实现SHA-1算法的示例代码:
```c++
#include <iostream>
#include <iomanip>
#include <sstream>
#include <string>
#include <cstring>
#include <cstdint>
// 左移循环移位
#define ROTL(x, n) (((x) << (n)) | ((x) >> (32 - (n))))
// SHA-1 常量
#define SHA1_K0 0x5A827999
#define SHA1_K1 0x6ED9EBA1
#define SHA1_K2 0x8F1BBCDC
#define SHA1_K3 0xCA62C1D6
// SHA-1 初始化向量
const std::uint32_t SHA1_IV[] = { 0x67452301, 0xEFCDAB89, 0x98BADCFE, 0x10325476, 0xC3D2E1F0 };
// SHA-1 消息填充
void sha1_pad(std::string& message) {
// 向消息尾部添加一个比特 1
message += '\x80';
// 添加 0 到 64 位填充,使消息长度满足模 512 余 448
std::size_t original_size = message.size();
std::size_t padding_size = (56 - (original_size % 64)) % 64;
message.resize(original_size + padding_size, '\0');
// 在消息尾部添加 64 位的原始消息长度
std::uint64_t message_bits = original_size * 8;
message.append(reinterpret_cast<const char*>(&message_bits), sizeof(message_bits));
}
// SHA-1 压缩函数
void sha1_compress(std::uint32_t* state, const std::uint8_t* block) {
// 初始化变量
std::uint32_t a = state[0];
std::uint32_t b = state[1];
std::uint32_t c = state[2];
std::uint32_t d = state[3];
std::uint32_t e = state[4];
std::uint32_t w[80];
// 将 16 个字分组成 80 个字
for (int i = 0; i < 16; i++) {
w[i] = (block[i * 4] << 24) | (block[i * 4 + 1] << 16) | (block[i * 4 + 2] << 8) | block[i * 4 + 3];
}
for (int i = 16; i < 80; i++) {
w[i] = ROTL(w[i - 3] ^ w[i - 8] ^ w[i - 14] ^ w[i - 16], 1);
}
// SHA-1 主循环
for (int i = 0; i < 80; i++) {
std::uint32_t f, k;
if (i < 20) {
f = (b & c) | ((~b) & d);
k = SHA1_K0;
} else if (i < 40) {
f = b ^ c ^ d;
k = SHA1_K1;
} else if (i < 60) {
f = (b & c) | (b & d) | (c & d);
k = SHA1_K2;
} else {
f = b ^ c ^ d;
k = SHA1_K3;
}
std::uint32_t temp = ROTL(a, 5) + f + e + k + w[i];
e = d;
d = c;
c = ROTL(b, 30);
b = a;
a = temp;
}
// 更新状态
state[0] += a;
state[1] += b;
state[2] += c;
state[3] += d;
state[4] += e;
}
// 计算 SHA-1 哈希值
std::string sha1(const std::string& message) {
// 初始化状态
std::uint32_t state[5];
std::memcpy(state, SHA1_IV, sizeof(SHA1_IV));
// 对消息进行填充
std::string padded_message = message;
sha1_pad(padded_message);
// 对填充后的消息进行压缩
for (std::size_t i = 0; i < padded_message.size(); i += 64) {
sha1_compress(state, reinterpret_cast<const std::uint8_t*>(&padded_message[i]));
}
// 将结果转换为十六进制字符串
std::ostringstream oss;
oss << std::hex << std::setfill('0');
for (int i = 0; i < 5; i++) {
oss << std::setw(8) << state[i];
}
return oss.str();
}
// 测试
int main() {
std::cout << sha1("Hello, world!") << std::endl; // 0x7b502c3a1f48c860e3c0feeb6a1c9a22d1aee6cb
return 0;
}
```
在该实现中,`sha1()` 函数接受一个字符串作为输入,并返回该字符串的 SHA-1 哈希值。哈希值以十六进制字符串的形式返回。
阅读全文
相关推荐














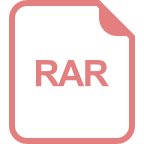


