python图书管理系统使用csv保存
时间: 2023-07-20 08:19:06 浏览: 121
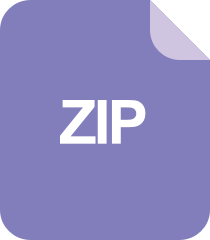
图书管理系统 Python实现
使用CSV文件保存数据是一种简单而有效的方法,以下是一些实现Python图书管理系统使用CSV文件保存数据的步骤:
1. 导入csv模块:在Python中使用csv模块,需要先导入它。
```python
import csv
```
2. 创建CSV文件:使用csv.writer()函数创建一个CSV文件,并将图书信息写入文件中。
```python
with open('books.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Book Title', 'Author', 'Publisher', 'ISBN', 'Status'])
writer.writerow(['The Alchemist', 'Paulo Coelho', 'HarperCollins', '9780061122415', 'Available'])
writer.writerow(['The Catcher in the Rye', 'J.D. Salinger', 'Little, Brown and Company', '9780316769488', 'Checked Out'])
```
3. 读取CSV文件:使用csv.reader()函数读取CSV文件中的图书信息。
```python
with open('books.csv', mode='r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
```
4. 更新CSV文件:使用csv.writer()函数更新CSV文件中的图书信息。
```python
with open('books.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Book Title', 'Author', 'Publisher', 'ISBN', 'Status'])
writer.writerow(['The Alchemist', 'Paulo Coelho', 'HarperCollins', '9780061122415', 'Available'])
writer.writerow(['The Catcher in the Rye', 'J.D. Salinger', 'Little, Brown and Company', '9780316769488', 'Checked Out'])
# Update the status of 'The Catcher in the Rye'
writer.writerow(['The Catcher in the Rye', 'J.D. Salinger', 'Little, Brown and Company', '9780316769488', 'Available'])
```
以上是一些实现Python图书管理系统使用CSV文件保存数据的步骤,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
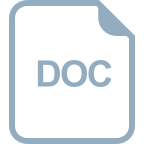
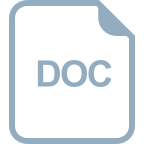


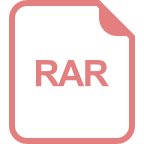
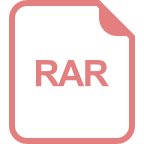
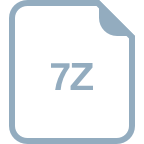
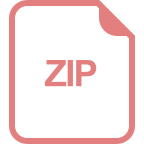
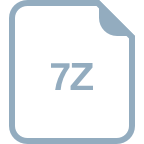
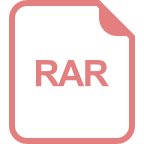
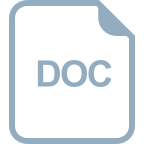
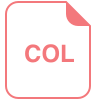





