帮我写一段Python代码,使用xgb回归进行预测,并输出拟合效果,可视化拟合结果
时间: 2023-06-18 07:05:22 浏览: 89
好的,以下是使用xgboost进行回归预测的Python代码:
```python
# 导入需要的库
import pandas as pd
import numpy as np
import xgboost as xgb
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# 读取数据
data = pd.read_csv('path/to/data.csv')
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.iloc[:, :-1], data.iloc[:, -1], test_size=0.2, random_state=42)
# 模型训练
params = {'objective': 'reg:squarederror', 'eval_metric': 'rmse'}
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test)
model = xgb.train(params, dtrain)
# 预测并输出拟合效果
y_pred = model.predict(dtest)
print('RMSE:', mean_squared_error(y_test, y_pred, squared=False))
# 可视化拟合结果
plt.scatter(y_test, y_pred)
plt.plot([y_test.min(), y_test.max()], [y_test.min(), y_test.max()], 'k--', lw=4)
plt.xlabel('True Values')
plt.ylabel('Predictions')
plt.show()
```
其中,需要替换的部分包括:
- `path/to/data.csv`:数据文件路径
- 数据集中的最后一列是因变量,其余列是自变量
- 可以根据需要调整`train_test_split`中的`test_size`参数来调整测试集大小
如果需要更进一步的模型调参和优化,可以使用网格搜索等方法。
相关推荐
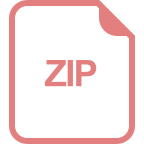
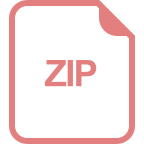














