用vhdl语言实现以下流程:书写一个彩灯控制器,先让8盏灯全灭,然后依次点亮,后让8盏灯全亮,然后依次熄灭,最后让8盏灯全亮全灭重复四次
时间: 2023-10-09 09:08:53 浏览: 62
好的,以下是一个简单的实现方案:
```vhdl
entity led_controller is
port (
clk : in std_logic; -- 时钟信号
rst : in std_logic; -- 复位信号
led : out std_logic_vector(7 downto 0) -- 控制8盏灯的信号
);
end entity;
architecture rtl of led_controller is
signal count : unsigned(2 downto 0) := (others => '0'); -- 计数器,用于延时
signal state : std_logic_vector(3 downto 0) := "0000"; -- 状态机状态
begin
process (clk)
begin
if rising_edge(clk) then
if rst = '1' then
-- 复位状态
count <= (others => '0');
state <= "0000";
led <= (others => '0');
else
-- 根据状态机状态控制灯的状态
case state is
when "0000" =>
-- 全灭状态
if count = 7 then
count <= (others => '0');
state <= "0001";
else
count <= count + 1;
end if;
led <= (others => '0');
when "0001" =>
-- 依次点亮
if count = 7 then
count <= (others => '0');
state <= "0010";
else
count <= count + 1;
end if;
led(count) <= '1';
when "0010" =>
-- 全亮状态
if count = 7 then
count <= (others => '0');
state <= "0011";
else
count <= count + 1;
end if;
led <= (others => '1');
when "0011" =>
-- 依次熄灭
if count = 7 then
count <= (others => '0');
state <= "0000";
else
count <= count + 1;
end if;
led(count) <= '0';
when others =>
null;
end case;
end if;
end if;
end process;
end architecture;
```
这个控制器包含一个简单的状态机,控制8盏灯的状态。当复位信号 `rst` 为高电平时,计数器 `count` 和状态机状态 `state` 都会被复位,同时所有灯都会被关闭。
在每一个时钟周期中,根据状态机状态 `state` 控制灯的状态。在状态 "0000" 中,所有灯都会被关闭,计数器 `count` 会逐渐增加,等到 `count` 达到 7 后,状态机会切换到状态 "0001",依次点亮8盏灯。当所有灯都点亮后,状态机会切换到状态 "0010",所有灯都会被点亮。然后状态机会切换到状态 "0011",依次熄灭8盏灯。最后状态机会切换回状态 "0000",重复上述过程。这样控制器就会不断循环地让8盏灯全灭、依次点亮、全亮、依次熄灭,循环四次后重新开始。
相关推荐
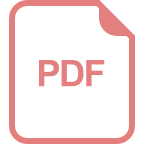
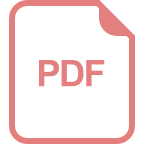
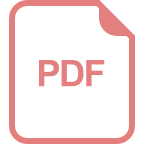














