写一个用pytorch实现可视化卷积神经网络的代码
时间: 2023-12-18 15:03:42 浏览: 27
你可以使用PyTorch中的torchvision库中的功能来可视化卷积神经网络,下面是一个简单的示例代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
import matplotlib.pyplot as plt
# 加载预训练的ResNet18网络
net = models.resnet18(pretrained=True)
# 将所有参数设置为不需要梯度
for param in net.parameters():
param.requires_grad = False
# 获取卷积层
conv_layers = []
for module in net.modules():
if isinstance(module, nn.Conv2d):
conv_layers.append(module)
# 取出其中一层的卷积核
conv_layer = conv_layers[1]
print(conv_layer)
# 定义预处理图像的方法
preprocess = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
# 加载一张图片并进行预处理
img_path = './test.jpg'
img = Image.open(img_path)
img_tensor = preprocess(img).unsqueeze(0)
# 将图像输入卷积层并输出feature map
output = conv_layer(img_tensor)
output = output.squeeze(0)
print(output.shape)
# 可视化feature map
fig, axarr = plt.subplots(3, 3, figsize=(10, 10))
for i in range(3):
for j in range(3):
axarr[i, j].imshow(output[i*3+j].data.cpu().numpy(), cmap='gray')
plt.show()
```
如果你想要可视化其他的卷积层,只需更改conv_layers中的索引即可。同时,请确保你已经安装了PyTorch和Matplotlib库。
相关推荐
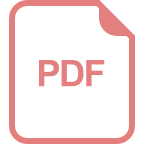
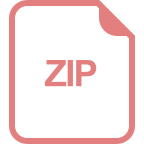
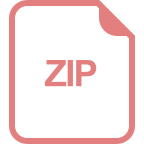
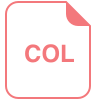
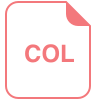












