SpringBoot在控制层接收前端传入的word文件,在服务层将word文件打开并调用数据库映射层将word文件中内容添加到数据库表中
时间: 2024-11-03 13:18:21 浏览: 26
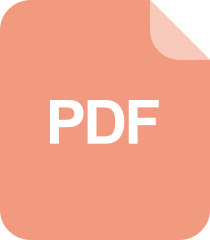
PHP执行SQL文件并将SQL文件导入到数据库

在Spring Boot应用中,处理Word文档的操作通常涉及到几个步骤:接收到文件、解析文件内容、操作数据库。由于Word文档的内容不是直接可以序列化的,通常需要借助第三方库如Apache POI来读取Word内容。以下是一个简化版的示例步骤:
1. **前端发送文件**:前端通过POST请求将Word文件作为二进制数据传递到后端。
```html
<form action="/process-word" method="post" enctype="multipart/form-data">
<input type="file" name="wordFile" accept=".docx" />
<button type="submit">上传</button>
</form>
```
2. **控制层接收文件**:在Spring Boot的Controller中,使用`@PostMapping`接收并保存文件。
```java
import org.springframework.web.multipart.MultipartFile;
@PostMapping("/process-word")
public ResponseEntity<?> processWordFile(@RequestParam("wordFile") MultipartFile wordFile) {
// ... 处理文件
if (wordFile.isEmpty()) {
return ResponseEntity.badRequest().body("文件为空");
}
try {
// 将文件保存到服务器某个目录
String filePath = saveFileToServer(wordFile);
// ... 继续处理
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件处理错误");
}
}
```
3. **服务层读取并解析Word内容**:使用Apache POI库来读取Word内容,这一步通常比较复杂,因为Word文档结构可能非常复杂。
```java
import org.apache.poi.xwpf.usermodel.*;
// ...
public String readWordContent(String filePath) throws IOException {
FileInputStream fis = new FileInputStream(filePath);
XWPFDocument doc = new XWPFDocument(fis);
StringBuilder contentBuilder = new StringBuilder();
for (XWPFParagraph para : doc.getParagraphs()) {
contentBuilder.append(para.getText());
}
fis.close();
return contentBuilder.toString();
}
// 调用这个方法来获取Word内容
String wordContent = readWordContent(filePath);
```
4. **数据库映射层操作**:将Word内容转化为数据库可以接受的数据结构,然后插入数据库。这里假设你有一个对应的实体类和Repository。
```java
public class WordDataEntity {
private String content;
// ... 省略其他字段
public String getContent() { ... }
public void setContent(String content) { ... }
}
@Autowired
private WordDataRepository wordDataRepository;
public void insertWordContentIntoDB(String content) {
WordDataEntity data = new WordDataEntity();
data.setContent(content);
wordDataRepository.save(data);
}
```
5. **整合整个流程**:在`processWordFile`方法中完成上述所有操作,然后返回响应结果。
注意:处理Word文件通常比处理文本文件更复杂,因为Word可能含有图片、表格等非纯文本内容。此外,操作Word文档最好在一个单独的线程或进程中进行,以免阻塞主线程。
阅读全文
相关推荐
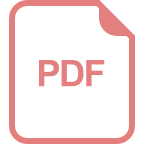
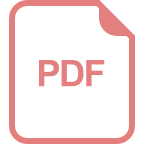
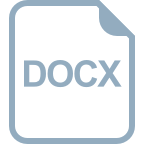
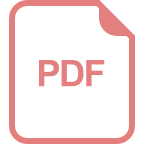
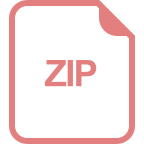
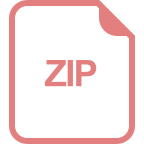
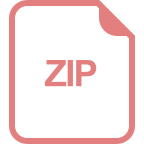
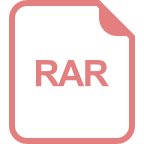
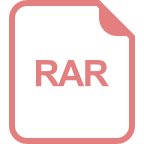
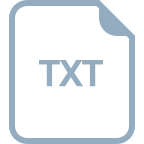
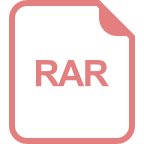
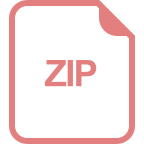
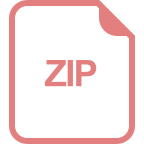
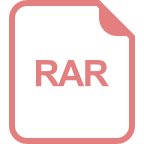
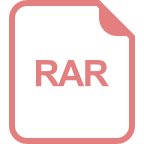
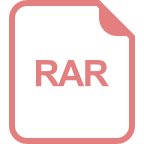