vue 写一个大数据可视化
时间: 2024-01-20 19:03:24 浏览: 24
大数据可视化是一项比较复杂的工作,需要结合数据处理、图表绘制等技术。Vue 作为一款流行的前端框架,可以很好地配合其他工具和库进行大数据可视化的开发。
下面提供一个简单的大数据可视化案例,仅供参考:
1. 准备数据
首先需要准备一些数据,可以从后台接口或者数据库中获取,这里我们使用 mock.js 来模拟数据,代码如下:
```js
import Mock from 'mockjs'
Mock.mock('/api/data', {
'data|10-50': [
{
'name|+1': ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'value|1000-5000': 1
}
]
})
```
2. 绘制柱状图
使用 echarts.js 绘制柱状图,代码如下:
```html
<template>
<div class="chart-container">
<div ref="chart" class="chart"></div>
</div>
</template>
<script>
import axios from 'axios'
import echarts from 'echarts'
export default {
data() {
return {
chart: null
}
},
mounted() {
axios.get('/api/data').then(res => {
const data = res.data.data
this.chart = echarts.init(this.$refs.chart)
this.chart.setOption({
xAxis: {
type: 'category',
data: data.map(item => item.name)
},
yAxis: {
type: 'value'
},
series: [{
data: data.map(item => item.value),
type: 'bar'
}]
})
})
},
beforeDestroy() {
if (this.chart) {
this.chart.dispose()
this.chart = null
}
}
}
</script>
<style scoped>
.chart-container {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.chart {
width: 800px;
height: 600px;
}
</style>
```
上面的代码中,我们使用了 axios 来获取数据,然后使用 echarts.js 绘制柱状图。在组件销毁前需要手动销毁 echarts 实例。
3. 绘制散点图
同样使用 echarts.js 绘制散点图,代码如下:
```html
<template>
<div class="chart-container">
<div ref="chart" class="chart"></div>
</div>
</template>
<script>
import axios from 'axios'
import echarts from 'echarts'
export default {
data() {
return {
chart: null
}
},
mounted() {
axios.get('/api/data').then(res => {
const data = res.data.data
this.chart = echarts.init(this.$refs.chart)
this.chart.setOption({
xAxis: {},
yAxis: {},
series: [{
data: data.map(item => [item.name, item.value]),
type: 'scatter'
}]
})
})
},
beforeDestroy() {
if (this.chart) {
this.chart.dispose()
this.chart = null
}
}
}
</script>
<style scoped>
.chart-container {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.chart {
width: 800px;
height: 600px;
}
</style>
```
上面的代码中,我们同样使用了 axios 来获取数据,然后使用 echarts.js 绘制散点图。
以上只是一个简单的示例,大数据可视化需要根据实际需求进行更加复杂的处理和绘制。
相关推荐
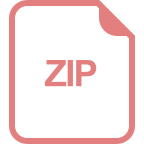
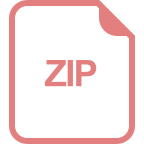
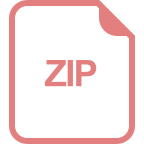
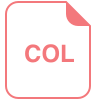
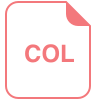
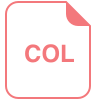
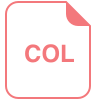
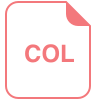









