、利用python和必要的库,实时监测计算机CPU负荷、内存占用率、温度
时间: 2023-07-10 11:13:58 浏览: 105
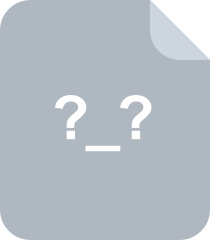
python 实时监控当前项目进程cpu使用率 内存使用率
可以利用Python中的psutil库来实现对计算机CPU负荷、内存占用率、温度的实时监测。具体实现方法如下:
首先,需要安装psutil库,可以通过以下命令来安装:
```
pip install psutil
```
然后,可以通过以下代码来实现对CPU负荷、内存占用率、温度的实时监测:
```python
import psutil
# 获取CPU使用率
cpu_percent = psutil.cpu_percent(interval=1)
# 获取内存使用率
mem = psutil.virtual_memory()
mem_percent = mem.percent
# 获取温度
temp = psutil.sensors_temperatures()
temp = temp.get('coretemp')[0].current
print('CPU使用率:{}%'.format(cpu_percent))
print('内存使用率:{}%'.format(mem_percent))
print('CPU温度:{}℃'.format(temp))
```
这段代码中,通过psutil库的cpu_percent()方法获取CPU使用率,virtual_memory()方法获取内存使用率,sensors_temperatures()方法获取温度,然后将获取到的数据打印输出即可。
其中,interval参数表示获取CPU使用率的时间间隔,单位为秒。在这里我们设置为1秒,即每隔1秒获取一次CPU使用率。
阅读全文
相关推荐
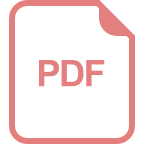




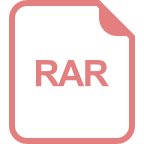
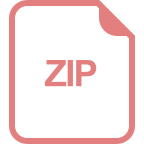
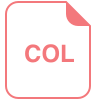
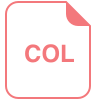
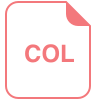
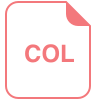
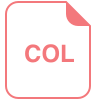
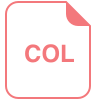
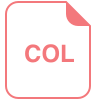
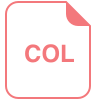
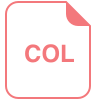
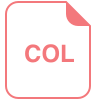