在RecycleView实现流式布局,FlexBoxLayoutManager布局超过一行时,显示内容为省略按钮
时间: 2024-03-24 12:36:39 浏览: 131
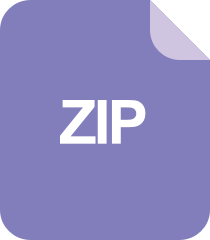
RecyclerView流式布局
如果您想在RecyclerView的FlexBoxLayoutManager布局中实现当行数超出一行时显示省略按钮的效果,可以通过以下步骤来实现:
1. 首先,定义一个自定义的FlexBoxLayoutManager,用于监听RecyclerView的滚动事件。
```java
public class CustomFlexBoxLayoutManager extends FlexboxLayoutManager {
private boolean isNeedEllipsis = false;
public CustomFlexBoxLayoutManager(Context context) {
super(context);
}
public CustomFlexBoxLayoutManager(Context context, int flexDirection) {
super(context, flexDirection);
}
public CustomFlexBoxLayoutManager(Context context, int flexDirection, int flexWrap) {
super(context, flexDirection, flexWrap);
}
@Override
public void onLayoutCompleted(RecyclerView.State state) {
super.onLayoutCompleted(state);
checkIfNeedEllipsis();
}
@Override
public void onScrollStateChanged(int state) {
super.onScrollStateChanged(state);
checkIfNeedEllipsis();
}
private void checkIfNeedEllipsis() {
int totalWidth = getWidth() - getPaddingLeft() - getPaddingRight();
int totalHeight = getHeight() - getPaddingTop() - getPaddingBottom();
int lineWidth = 0;
int lineHeight = 0;
int lineCount = 1;
isNeedEllipsis = false;
for (int i = 0; i < getItemCount(); i++) {
View view = findViewByPosition(i);
if (view != null) {
int width = getDecoratedMeasuredWidth(view);
int height = getDecoratedMeasuredHeight(view);
if (lineWidth + width > totalWidth) {
lineWidth = width;
lineHeight = height;
lineCount++;
if (lineCount > 1) {
isNeedEllipsis = true;
break;
}
} else {
lineWidth += width;
lineHeight = Math.max(lineHeight, height);
}
}
}
if (isNeedEllipsis) {
// 当超出一行时,显示省略按钮
// TODO: 显示省略按钮
} else {
// 当不超出一行时,隐藏省略按钮
// TODO: 隐藏省略按钮
}
}
public boolean isNeedEllipsis() {
return isNeedEllipsis;
}
}
```
2. 在RecyclerView中设置自定义的FlexBoxLayoutManager,并设置一个监听器来监听省略按钮的点击事件。
```java
RecyclerView recyclerView = findViewById(R.id.recyclerView);
CustomFlexBoxLayoutManager layoutManager = new CustomFlexBoxLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
layoutManager.setOnEllipsisButtonClickListener(new OnEllipsisButtonClickListener() {
@Override
public void onEllipsisButtonClick() {
// 处理省略按钮的点击事件
// TODO: 处理省略按钮的点击事件
}
});
```
3. 在CustomFlexBoxLayoutManager的checkIfNeedEllipsis()方法中,通过遍历所有的item来计算当前行数,判断当前行数是否超出一行。如果超出,则显示省略按钮,否则隐藏省略按钮。在显示省略按钮时,您可以使用PopupWindow或者Dialog等方式来实现。在隐藏省略按钮时,需要将省略按钮隐藏掉,以免影响用户体验。
4. 最后,您还可以通过设置RecyclerView的padding来控制省略按钮的显示位置。在显示省略按钮时,您可以将省略按钮添加到RecyclerView的父布局中,以便在滚动RecyclerView时不会被遮挡。
```java
recyclerView.setPadding(0, 0, ellipsisButtonWidth, 0);
```
其中,ellipsisButtonWidth是省略按钮的宽度。
阅读全文
相关推荐
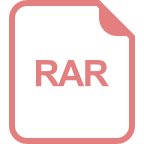
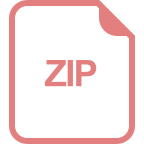
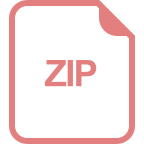
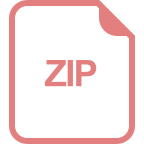
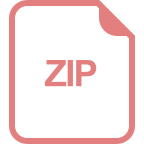
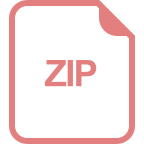
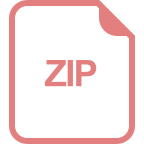
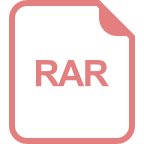
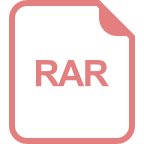
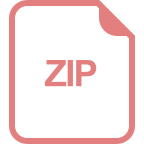
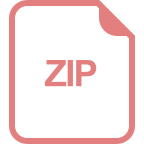





