c++面向对象编程示例,要求包含多个类
时间: 2023-12-30 20:04:48 浏览: 27
以下是一个基于面向对象编程的示例,包含三个类:Person、Student和Teacher。
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
def study(self):
print(f"{self.name} is studying.")
class Teacher(Person):
def __init__(self, name, age, subject):
super().__init__(name, age)
self.subject = subject
def teach(self):
print(f"{self.name} is teaching {self.subject}.")
```
在这个示例中,Person是一个基类,Student和Teacher是两个派生类。Person类有两个属性(name和age)和一个方法(say_hello),表示一个普通人。Student类继承了Person类,并且添加了一个新的属性(student_id)和一个新的方法(study),表示一个学生。Teacher类同样继承了Person类,并且添加了一个新的属性(subject)和一个新的方法(teach),表示一个老师。
下面是如何使用这些类创建对象并调用它们的方法的示例:
```python
person1 = Person("Alice", 25)
person1.say_hello()
student1 = Student("Bob", 20, "123456")
student1.say_hello()
student1.study()
teacher1 = Teacher("Charlie", 30, "Math")
teacher1.say_hello()
teacher1.teach()
```
输出:
```
Hello, my name is Alice and I am 25 years old.
Hello, my name is Bob and I am 20 years old.
Bob is studying.
Hello, my name is Charlie and I am 30 years old.
Charlie is teaching Math.
```
通过这个示例,我们可以看到面向对象编程的优势:我们可以创建不同类型的对象,并且这些对象可以具有不同的属性和方法,但是它们都可以基于同一个类来创建。这使得我们的代码更加模块化和可重用,同时也更加易于维护和扩展。
相关推荐
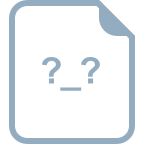
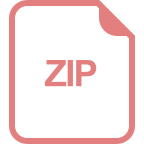
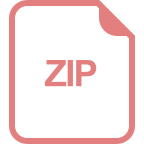














