用vue写一个背单词系统
时间: 2023-04-08 09:04:01 浏览: 117
好的,我可以回答这个问题。使用Vue可以很方便地构建一个背单词系统。您可以使用Vue CLI来创建一个新的Vue项目,然后使用Vue Router来管理不同页面之间的导航。您可以使用Vue组件来构建不同的页面和功能,例如单词列表、单词卡片、测试页面等。您还可以使用Vue的生命周期钩子函数来管理组件的状态和行为。最后,您可以使用Vue的数据绑定和事件处理机制来实现用户与系统的交互。希望这可以帮助您开始构建一个背单词系统。
相关问题
使用vue写一个背单词代码
好的,我可以回答这个问题。以下是一个使用Vue编写的背单词代码的示例:
```
<template>
<div>
<h1>{{ currentWord }}</h1>
<button @click="showTranslation = !showTranslation">
{{ showTranslation ? 'Hide' : 'Show' }} Translation
</button>
<p v-if="showTranslation">{{ currentTranslation }}</p>
<button @click="nextWord">Next Word</button>
</div>
</template>
<script>
export default {
data() {
return {
words: [
{ word: 'apple', translation: '苹果' },
{ word: 'banana', translation: '香蕉' },
{ word: 'orange', translation: '橙子' },
],
currentIndex: 0,
showTranslation: false,
};
},
computed: {
currentWord() {
return this.words[this.currentIndex].word;
},
currentTranslation() {
return this.words[this.currentIndex].translation;
},
},
methods: {
nextWord() {
this.currentIndex = (this.currentIndex + 1) % this.words.length;
this.showTranslation = false;
},
},
};
</script>
```
这个代码使用了Vue的模板语法和组件化开发方式,实现了一个简单的背单词应用。用户可以点击“Next Word”按钮来切换当前显示的单词,点击“Show Translation”按钮来显示或隐藏当前单词的翻译。
用vue写一个背单词功能
好的,我可以回答这个问题。使用Vue可以很方便地实现背单词功能,可以通过Vue的组件化思想,将单词列表、单词卡片、单词测试等功能拆分成不同的组件,便于管理和维护。同时,可以利用Vue的数据绑定和计算属性等特性,实现单词的添加、删除、修改、查询等操作。另外,可以结合第三方API,如有道词典API等,实现单词的翻译和发音等功能,提高用户体验。
相关推荐
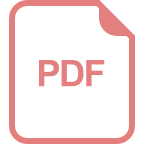
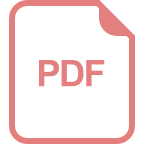











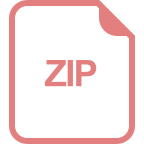