java8两个list集合根据某个字段取交集
时间: 2024-02-03 08:11:43 浏览: 37
可以使用Java 8的Stream API来实现两个List集合根据某个字段取交集。
假设有两个List集合,每个集合都有一个Person对象,其中Person对象有一个id属性,我们需要根据id属性来取交集,可以按照以下步骤操作:
1. 将两个List集合转换成Map,其中Map的key为id,value为Person对象。
```java
Map<Integer, Person> map1 = list1.stream().collect(Collectors.toMap(Person::getId, Function.identity()));
Map<Integer, Person> map2 = list2.stream().collect(Collectors.toMap(Person::getId, Function.identity()));
```
2. 使用map1的keySet()与map2的keySet()取交集,得到交集的id集合。
```java
Set<Integer> intersection = map1.keySet().stream().filter(map2::containsKey).collect(Collectors.toSet());
```
3. 根据交集的id集合从map1中获取Person对象集合。
```java
List<Person> result = map1.entrySet().stream().filter(entry -> intersection.contains(entry.getKey())).map(Map.Entry::getValue).collect(Collectors.toList());
```
完整代码如下:
```java
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Person> list1 = Arrays.asList(new Person(1, "Tom"), new Person(2, "Jerry"), new Person(3, "Mike"));
List<Person> list2 = Arrays.asList(new Person(2, "Jerry"), new Person(3, "Mike"), new Person(4, "John"));
Map<Integer, Person> map1 = list1.stream().collect(Collectors.toMap(Person::getId, Function.identity()));
Map<Integer, Person> map2 = list2.stream().collect(Collectors.toMap(Person::getId, Function.identity()));
Set<Integer> intersection = map1.keySet().stream().filter(map2::containsKey).collect(Collectors.toSet());
List<Person> result = map1.entrySet().stream().filter(entry -> intersection.contains(entry.getKey())).map(Map.Entry::getValue).collect(Collectors.toList());
System.out.println(result);
}
}
class Person {
private int id;
private String name;
public Person(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Person{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
```
相关推荐
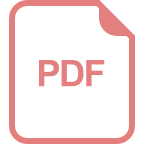
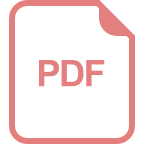














