消灭星星键盘和鼠标控制选择星星并消除的代码c++
时间: 2024-03-23 12:43:10 浏览: 91
以下是一个简单的 C++ 代码示例,可以实现消灭星星游戏的功能,使用键盘和鼠标来控制选择星星并消除。
```
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <vector>
using namespace std;
const int ROWS = 10;
const int COLS = 10;
const int COLORS = 5;
int board[ROWS][COLS];
// 在棋盘上随机生成星星
void generateBoard() {
srand(time(NULL));
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = rand() % COLORS + 1;
}
}
}
// 显示棋盘上的星星
void displayBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
// 处理点击事件
void handleClick(int row, int col) {
int color = board[row][col];
vector<pair<int, int>> starsToRemove;
// 找出相邻的同色星星
findAdjacentStars(row, col, color, starsToRemove);
// 如果找到了相邻的星星,就消除它们
if (starsToRemove.size() >= 3) {
removeStars(starsToRemove);
}
}
// 找出相邻的同色星星
void findAdjacentStars(int row, int col, int color, vector<pair<int, int>>& starsToRemove) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
return;
}
if (board[row][col] != color) {
return;
}
for (auto star : starsToRemove) {
if (star.first == row && star.second == col) {
return;
}
}
starsToRemove.push_back(make_pair(row, col));
findAdjacentStars(row - 1, col, color, starsToRemove);
findAdjacentStars(row + 1, col, color, starsToRemove);
findAdjacentStars(row, col - 1, color, starsToRemove);
findAdjacentStars(row, col + 1, color, starsToRemove);
}
// 消除星星
void removeStars(vector<pair<int, int>>& starsToRemove) {
for (auto star : starsToRemove) {
board[star.first][star.second] = 0;
}
}
int main() {
generateBoard();
displayBoard();
// 模拟点击事件,选中第一行第一列的星星
handleClick(0, 0);
displayBoard();
return 0;
}
```
在以上代码中,`generateBoard` 函数会在棋盘上随机生成星星,`displayBoard` 函数会显示棋盘上的星星。当玩家点击一个星星时,`handleClick` 函数会找出相邻的同色星星,并将它们消除。
你可以在代码中自定义棋盘的行数、列数和颜色数,以及定义更多的函数来实现更多的功能。
阅读全文
相关推荐
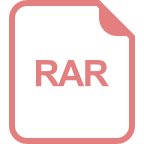
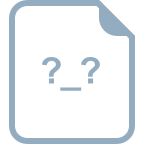
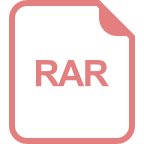
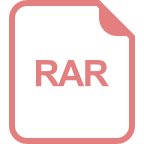
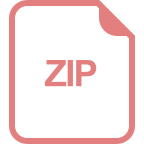
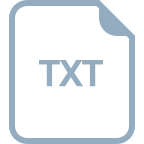
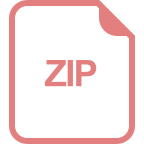
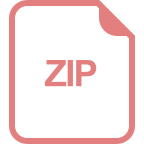
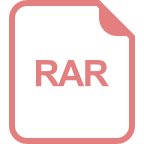
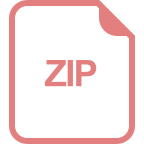
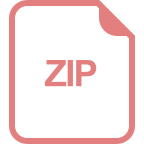
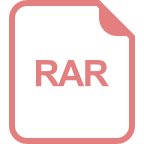
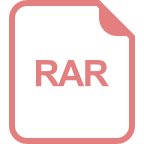
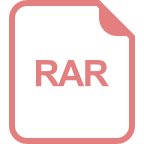
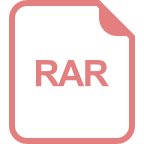
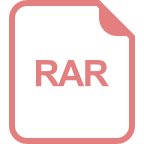