List<DevPortReportEsDTO> list = handleEsData(pageResult1); //List<DevPortReportEsDTO> list = this.extEsResponse(pageResult1); log.info("链路组流量报表es查询返回为:{}", JSONUtil.toJsonStr(list)); log.info("EsServiceBiz.getRelayLinkReportByEs#linkGroupDevPortMap计算前:{}", JSONUtil.toJsonStr(linkGroupDevPortMap)); // es查询返回的数据组成map,key为devId+portName,value为DevPortReportEsDTO对象 Map<String, DevPortReportEsDTO> reportEsDTOMap = list.stream() .collect(Collectors.toMap(reportEsDTO -> reportEsDTO.getMo_id() + reportEsDTO.getObject_id(), reportEsDTO -> reportEsDTO)); // 遍历链路组下包含的端口 Map<String, List<PortReportResultVo>> relayLinkAndPortMap = relayLinkAndPortListMap.entrySet().stream() .collect(Collectors.toMap( Map.Entry::getKey, entry -> entry.getValue().stream().map(trDevportVO -> { String tempKey = trDevportVO.getDevId() + trDevportVO.getPortName(); DevPortReportEsDTO reportEsDTO = reportEsDTOMap.get(tempKey); PortReportResultVo reportResultVo = new PortReportResultVo(); BeanUtil.copyProperties(trDevportVO, reportResultVo); reportResultVo.setPortBandwidth(trDevportVO.getBandwidth()); BeanUtil.copyProperties(reportEsDTO, reportResultVo); return reportResultVo; }).collect(Collectors.toList()) )); 优化这段代码 优化这段代码
时间: 2024-01-22 15:21:33 浏览: 81
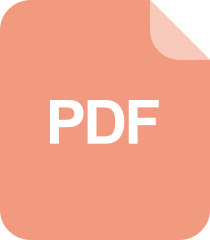
java将String字符串转换为List<Long>类型实例方法
首先,可以将两个 `collect` 操作合并为一个,避免了多次迭代集合的开销。可以这样写:
```
Map<String, List<PortReportResultVo>> relayLinkAndPortMap = relayLinkAndPortListMap.entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> {
return entry.getValue().stream().map(trDevportVO -> {
String tempKey = trDevportVO.getDevId() + trDevportVO.getPortName();
DevPortReportEsDTO reportEsDTO = reportEsDTOMap.get(tempKey);
PortReportResultVo reportResultVo = new PortReportResultVo();
BeanUtil.copyProperties(trDevportVO, reportResultVo);
reportResultVo.setPortBandwidth(trDevportVO.getBandwidth());
BeanUtil.copyProperties(reportEsDTO, reportResultVo);
return reportResultVo;
}).collect(Collectors.toList());
}
));
```
其次,可以将 `reportEsDTOMap` 的计算放在 `handleEsData` 方法中完成。这样可以提高代码的复用性,也可以避免在每次调用 `handleEsData` 时都重复计算 `reportEsDTOMap` 的开销。例如:
```
private List<DevPortReportEsDTO> handleEsData(PageResult<JSONObject> pageResult) {
List<DevPortReportEsDTO> list = this.extEsResponse(pageResult);
Map<String, DevPortReportEsDTO> reportEsDTOMap = list.stream()
.collect(Collectors.toMap(
reportEsDTO -> reportEsDTO.getMo_id() + reportEsDTO.getObject_id(),
reportEsDTO -> reportEsDTO
));
return list;
}
```
最后,可以使用方法引用简化代码。例如:
```
Map<String, List<PortReportResultVo>> relayLinkAndPortMap = relayLinkAndPortListMap.entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> entry.getValue().stream().map(trDevportVO -> {
String tempKey = trDevportVO.getDevId() + trDevportVO.getPortName();
PortReportResultVo reportResultVo = new PortReportResultVo();
BeanUtil.copyProperties(trDevportVO, reportResultVo);
reportResultVo.setPortBandwidth(trDevportVO.getBandwidth());
BeanUtil.copyProperties(reportEsDTOMap.get(tempKey), reportResultVo);
return reportResultVo;
}).collect(Collectors.toList())
));
```
综上所述,优化后的代码如下所示:
```
List<DevPortReportEsDTO> list = handleEsData(pageResult1);
log.info("链路组流量报表es查询返回为:{}", JSONUtil.toJsonStr(list));
log.info("EsServiceBiz.getRelayLinkReportByEs#linkGroupDevPortMap计算前:{}", JSONUtil.toJsonStr(linkGroupDevPortMap));
Map<String, List<PortReportResultVo>> relayLinkAndPortMap = relayLinkAndPortListMap.entrySet().stream()
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> entry.getValue().stream().map(trDevportVO -> {
String tempKey = trDevportVO.getDevId() + trDevportVO.getPortName();
PortReportResultVo reportResultVo = new PortReportResultVo();
BeanUtil.copyProperties(trDevportVO, reportResultVo);
reportResultVo.setPortBandwidth(trDevportVO.getBandwidth());
BeanUtil.copyProperties(reportEsDTOMap.get(tempKey), reportResultVo);
return reportResultVo;
}).collect(Collectors.toList())
));
```
阅读全文
相关推荐
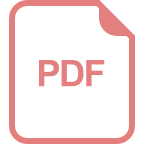
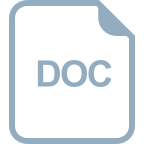

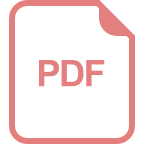
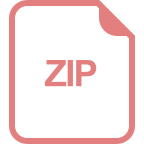
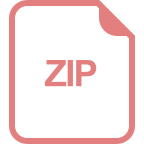
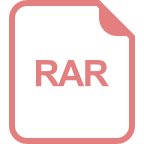
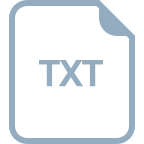
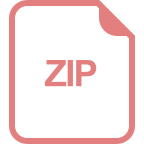
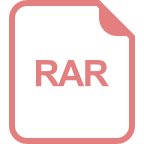
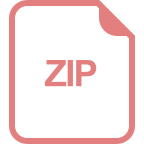
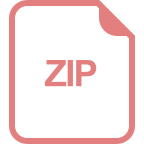
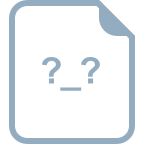
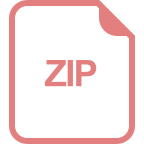
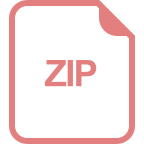