再创新一个结构体来实现两个结构体数据两两交换的话,新创建的那个结构体需要malloc吗?
时间: 2024-08-13 09:06:58 浏览: 55
为了实现两个结构体数据的两两交换,我们可以创建一个新的结构体,这个新结构体包含两个指向原始结构体变量的指针。在这种情况下,新结构体本身并不需要`malloc`动态分配内存,因为它并不存储额外的数据,只是作为一个容器来保存引用。
新结构体的设计可能如下:
```c
typedef struct {
struct TypeA *a;
struct TypeB *b;
} StructureExchange;
// 在需要交换的地方
StructureExchange *exchange = malloc(sizeof(StructureExchange)); // 如果TypeA和TypeB是动态分配的,这里需要malloc
exchange->a = &typeAInstance; // 假设typeAInstance是TypeA的实例
exchange->b = &typeBInstance; // 假设typeBInstance是TypeB的实例
// 交换后
TypeAInstance = exchange->b->data; // 假设TypeA和TypeB都有"data"成员用于交换
typeBInstance.data = exchange->a->data; // 类似的操作
// 释放交换用的结构体
free(exchange);
```
相关问题
原始有两个结构体,再创新一个结构体来实现三个个结构体数据两两交换的,从而达到原始结构体数据交换,新创建的那个结构体需要malloc吗?
当你有两个结构体需要彼此交换数据,你可以创建一个新的结构体来封装这两个原有结构体,并在新结构体中包含指向它们的指针。这样,新结构体并不一定需要使用`malloc()`来分配内存,除非你需要动态地创建这个结构体。如果两个原始结构体是静态存储或者栈上的,那么新结构体可以是静态的,只包含指针。
以下是一个简单的例子,假设我们有两个结构体`struct A`和`struct B`:
```c
typedef struct A {
int a;
char b;
} A;
typedef struct B {
float c;
char d;
} B;
// 新结构体,用于存储并交换A和B的数据
typedef struct CombinedStruct {
A* a_ptr;
B* b_ptr;
} CombinedStruct;
void swap(A* a1, A* a2) {
int temp = a1->a;
a1->a = a2->a;
a2->a = temp;
char temp_char = a1->b;
a1->b = a2->b;
a2->b = temp_char;
}
void swap(B* b1, B* b2) {
float temp = b1->c;
b1->c = b2->c;
b2->c = temp;
char temp_char = b1->d;
b1->d = b2->d;
b2->d = temp_char;
}
// 创建CombinedStruct,不需要malloc
CombinedStruct combined;
combined.a_ptr = &a1;
combined.b_ptr = &b1;
swap(combined.a_ptr, combined.b_ptr); // 在这里交换数据
// 如果需要动态创建,可以这样做(但不是必需的)
CombinedStruct* dynamic_combined = (CombinedStruct*)malloc(sizeof(CombinedStruct));
dynamic_combined->a_ptr = &a1;
dynamic_combined->b_ptr = &b1;
swap(dynamic_combined->a_ptr, dynamic_combined->b_ptr);
```
原始有两个结构体,再创新一个结构体来实现三个结构体数据两两交换的,从而达到原始结构体数据交换,新创建的那个结构体需要malloc吗?
当你有两个结构体需要彼此交换数据,但又不希望修改原始结构体,可以通过创建一个新的临时结构体来完成这个操作,这样可以避免直接对原始结构体的修改。新创建的结构体通常是动态分配内存(使用`malloc`)的,因为它的大小可能取决于原始结构体的类型,而不是固定的。
以下是一个简单的示例,假设我们有两个结构体`StructA`和`StructB`:
```c
#include <stdio.h>
#include <stdlib.h>
// 假设这两个结构体如下
struct StructA {
int a;
char b[20];
};
struct StructB {
float c;
char d[30];
};
// 创建一个临时结构体来存储数据
typedef struct {
union {
struct StructA a_data;
struct StructB b_data;
};
struct StructA* a; // 或者 struct StructB* b, 用于保存原始结构体指针
} TemporaryStruct;
// 功能函数,用于交换数据
void swapData(struct StructA* a1, struct StructB* b1, TemporaryStruct* temp) {
temp->a = a1;
temp->b_data.c = a1->a;
strcpy(temp->b_data.d, a1->b);
temp->a_data.a = b1->c;
strcpy(temp->a_data.b, b1->d);
}
int main() {
// 分配内存
struct StructA originalA = {1, "Original A"};
struct StructB originalB = {3.14, "Original B"};
TemporaryStruct* temp = malloc(sizeof(TemporaryStruct));
// 交换数据
swapData(&originalA, &originalB, temp);
// 现在你可以访问temp中的数据,原始结构体保持不变
printf("After swapping: A.a = %d, B.c = %.2f\n", temp->a_data.a, temp->b_data.c);
free(temp); // 必须释放临时结构体的内存
return 0;
}
阅读全文
相关推荐
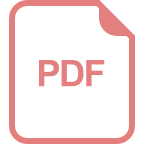
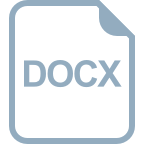
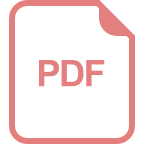
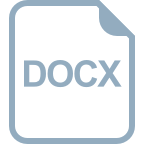
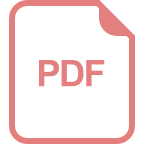
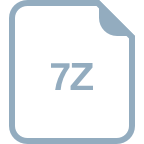
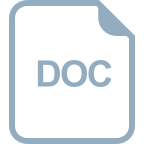









