分割字符串】 给定一个字符串:"itheima itcast boxuegu" 1、统计字符串内有多少个"it"字符 2、将字符串内的空格,全部替换为字符:"|" 3、并按照"|"进行字符串分割,得到列表
时间: 2023-12-10 20:03:02 浏览: 202
1. 统计字符串内有多少个"it"字符:
```python
str = "itheima itcast boxuegu"
count = str.count("it")
print(count) # 输出2
```
2. 将字符串内的空格,全部替换为字符:"|"
```python
str = "itheima itcast boxuegu"
new_str = str.replace(" ", "|")
print(new_str) # 输出"itheima|itcast|boxuegu"
```
3. 并按照"|"进行字符串分割,得到列表
```python
str = "itheima|itcast|boxuegu"
lst = str.split("|")
print(lst) # 输出["itheima", "itcast", "boxuegu"]
```
可以将以上三个步骤结合起来进行操作,代码如下:
```python
str = "itheima itcast boxuegu"
count = str.count("it")
new_str = str.replace(" ", "|")
lst = new_str.split("|")
print(count) # 输出2
print(new_str) # 输出"itheima|itcast|boxuegu"
print(lst) # 输出["itheima", "itcast", "boxuegu"]
```
相关问题
给定一个字符串:"itheima itcast boxuegu",完成以下操作并输出结果: 1. 统计字符串内有多少个"it"字符 2. 将字符串内的空格,全部替换为字符:"#" 3. 并按照"#"进行字符串分割,得到列表
首先,我们可以使用Python来逐项完成这些操作:
1. 统计"it"字符的数量:
```python
s = "itheima itcast boxuegu"
count = s.count('it')
print(f"字符串中有 {count} 个 'it' 字符")
```
2. 替换字符串中的所有空格为 "#":
```python
new_s = s.replace(' ', '#')
```
3. 按照 "#" 进行字符串分割:
```python
split_list = new_s.split('#')
print(f"分割后的列表:{split_list}")
```
将以上代码组合在一起:
```python
s = "itheima itcast boxuegu"
count = s.count('it')
new_s = s.replace(' ', '#')
split_list = new_s.split('#')
print(f"字符串中有 {count} 个 'it' 字符")
print(f"替换空格后的字符串:{new_s}")
print(f"分割后的列表:{split_list}")
```
执行这段代码后,你会看到统计结果、替换后的字符串以及分割后的列表。
要求:下列各题要求打印输出结果。 一,查找 定义字符串: mystr = hello world and itcast and itheima and python 按下列要求对mystr字符串进行操作,并将结果打印输出. 1.find( (1)查找and子串出现位置下标 (2
在Python中,可以使用`find()`函数来查找子串在字符串中的位置。对于给定的字符串`mystr = "hello world and itcast and itheima and python"`,我们可以按照您的要求进行操作:
1. 查找"and"子串出现的位置下标:
```python
mystr = "hello world and itcast and itheima and python"
index = mystr.find("and")
print(f"And子串第一次出现的位置是:{index}")
```
这里注意,`find()`返回的是子串首次出现的位置,如果没有找到则返回-1。
2. 查找"python"子串出现的位置下标:
```python
py_index = mystr.find("python")
print(f"Python子串出现的位置是:{py_index}")
```
同样,如果没找到会返回-1。
3. 如果想查找所有"and"子串出现的位置,可以循环查找并存储:
```python
all_indices = [mystr.find("and", i) for i in range(len(mystr))]
print("所有的'and'子串位置:", all_indices)
```
这将返回一个列表,包含每个"and"子串首次出现的所有索引。
阅读全文
相关推荐
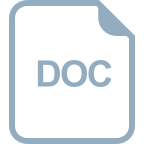
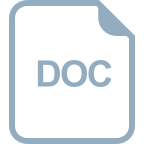
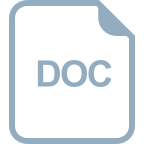
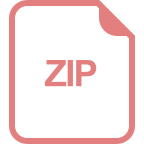
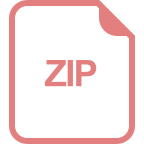
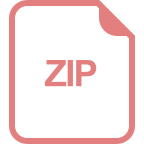










