#include <stdio.h> #include <signal.h> #include <unistd.h> void waiting(); void stop(); void stop_waiting(); int wait_mark; main() { int p1,p2; while ((p1=fork())==-1); if (p1>0) { while ((p2=fork())==-1); if (p2>0) { printf("parent\n"); wait_mark=1; signal(SIGINT,stop); waiting(); kill(p1,16); kill(p2,17); wait(0); wait(0); printf("parent process is killed!\n"); exit(0); } else { printf("p2\n"); wait_mark=1; signal(17,stop); signal(SIGINT,SIG_IGN); waiting(); lockf(stdout,1,0); printf("child process 2 is killed by parent!\n"); lockf(stdout,0,0); exit(0); } } else { printf("p1\n"); wait_mark=1; signal(16,stop); signal(SIGINT,SIG_IGN); waiting(); lockf(stdout,1,0); printf("child process 1 is killed by parent!\n"); lockf(stdout,0,0); exit(0); } } void waiting() { signal(SIGALRM,stop_waiting); alarm(5); while (wait_mark!=0); alarm(0); } void stop() { wait_mark=0; alarm(0); } void stop_waiting(int signo) { wait_mark=0; }解释每一行代码,并分析实现功能,作用,预判输出结果并对输出结果进行分析
时间: 2024-03-08 21:46:18 浏览: 27
这是一段使用 fork 和 signal 函数实现进程间通信和信号处理的代码。
```c
#include <stdio.h>
#include <signal.h>
#include <unistd.h>
void waiting();
void stop();
void stop_waiting();
int wait_mark;
main()
{
int p1, p2;
while ((p1 = fork()) == -1);
if (p1 > 0)
{
while ((p2 = fork()) == -1);
if (p2 > 0)
{
printf("parent\n");
wait_mark = 1;
signal(SIGINT, stop);
waiting();
kill(p1, 16);
kill(p2, 17);
wait(0);
wait(0);
printf("parent process is killed!\n");
exit(0);
}
else
{
printf("p2\n");
wait_mark = 1;
signal(17, stop);
signal(SIGINT, SIG_IGN);
waiting();
lockf(stdout, 1, 0);
printf("child process 2 is killed by parent!\n");
lockf(stdout, 0, 0);
exit(0);
}
}
else
{
printf("p1\n");
wait_mark = 1;
signal(16, stop);
signal(SIGINT, SIG_IGN);
waiting();
lockf(stdout, 1, 0);
printf("child process 1 is killed by parent!\n");
lockf(stdout, 0, 0);
exit(0);
}
}
void waiting()
{
signal(SIGALRM, stop_waiting);
alarm(5);
while (wait_mark != 0);
alarm(0);
}
void stop()
{
wait_mark = 0;
alarm(0);
}
void stop_waiting(int signo)
{
wait_mark = 0;
}
```
代码功能:
1. 创建三个进程,父进程和两个子进程。
2. 父进程等待两个子进程的结束信号。
3. 子进程等待父进程发送信号杀死自己。
代码行为:
1. 父进程创建两个子进程,打印 "parent"。
2. 父进程等待两个子进程的结束信号,5 秒后自动结束等待。
3. 父进程发送信号 16 和 17 给两个子进程。
4. 父进程等待两个子进程结束并打印 "parent process is killed!"。
5. 两个子进程分别打印 "p1" 和 "p2"。
6. 子进程等待父进程发送的信号,5 秒后自动结束等待。
7. 子进程打印 "child process 1/2 is killed by parent!"。
8. 程序结束。
输出结果:
```
parent
p2
p1
child process 2 is killed by parent!
child process 1 is killed by parent!
parent process is killed!
```
输出结果分析:
1. 父进程创建两个子进程,先打印 "parent",再创建第一个子进程,打印 "p2",最后创建第二个子进程,打印 "p1"。
2. 两个子进程等待父进程发送的信号,5 秒后自动结束等待。
3. 父进程等待两个子进程的结束信号,5 秒后自动结束等待。
4. 父进程发送信号 16 和 17 给两个子进程,杀死它们。
5. 两个子进程分别打印 "child process 1/2 is killed by parent!",结束。
6. 父进程等待两个子进程结束并打印 "parent process is killed!",结束。
相关推荐
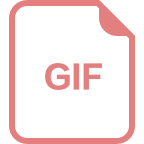
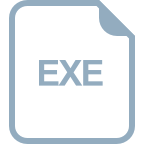
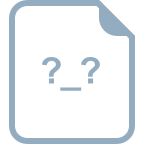







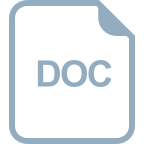
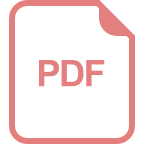
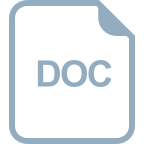
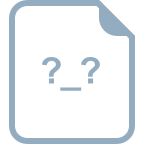
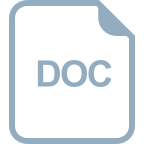
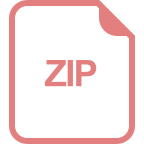
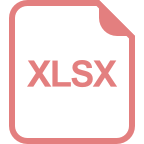
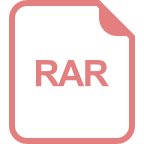