LocalTime localTime = now.toLocalTime(); //获取当前本机时间 例如2023-05-30 LocalDate localDate = now.toLocalDate(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); // 设置日期格式为 yyyy-MM-dd //获取数据库最近打卡的时间 2023-05-30 if (lastAttendance==null){ if (localTime.isBefore(LocalTime.of(9, 0))){ attendance.setType("上班签到"); attendance.setNumber(1); }else { attendance.setType("上班迟到"); attendance.setNumber(1); attendanceService.save(attendance); return "success"; } } String formattedDate = sdf.format(lastAttendance.getDate());
时间: 2024-02-14 11:05:49 浏览: 76
这段代码首先获取当前本机时间和日期,然后通过SimpleDateFormat将日期格式化为"yyyy-MM-dd"的字符串,接着获取数据库中最近一次打卡记录的日期并将其格式化为相同的字符串格式。如果最近一次打卡记录为null,则根据当前时间判断是签到还是迟到,并将打卡类型和打卡次数设置为1。如果最近一次打卡记录不为null,则判断是否是当天,如果是当天,则根据上次打卡类型和时间判断当前打卡类型和次数,并进行相应的设置。如果不是当天,则根据当前时间判断是签到还是迟到,并将打卡类型和打卡次数设置为1。
相关问题
LocalTime startTime1 = LocalTime.of(8, 0); // 第一个时间区间开始时间LocalTime endTime1 = LocalTime.of(18, 0); // 第一个时间区间结束时间LocalTime startTime2 = LocalTime.of(18, 0); // 第二个时间区间开始时间LocalTime endTime2 = LocalTime.of(8, 0); // 第二个时间区间结束时间LocalDateTime inputStartDateTime = LocalDateTime.of(LocalDate.now(), LocalTime.parse("13:30")); // 输入的时间段开始时间LocalDateTime inputEndDateTime = LocalDateTime.of(LocalDate.now(), LocalTime.parse("19:00")); // 输入的时间段结束时间// 判断是否跨夜if (inputStartDateTime.isAfter(inputEndDateTime)) { inputEndDateTime = inputEndDateTime.plusDays(1);}// 判断属于哪个时间区间if (inputStartDateTime.toLocalTime().compareTo(startTime1) >= 0 && inputEndDateTime.toLocalTime().compareTo(endTime1) <= 0) { System.out.println("属于第一个时间区间");} else if (inputStartDateTime.toLocalTime().compareTo(startTime2) >= 0 || inputEndDateTime.toLocalTime().compareTo(endTime2) <= 0) { System.out.println("属于第二个时间区间");} else { System.out.println("不属于任何时间区间");}
这段代码的问题在于对于第二个时间区间的判断条件不够准确。当输入的时间段跨越午夜时,即输入的开始时间晚于结束时间时,应该判断输入时间段是否在第二个时间区间中,而不是只判断输入结束时间是否在第二个时间区间中。因此,你可以将第二个判断条件改为:
```
else if (inputStartDateTime.toLocalTime().compareTo(startTime2) >= 0 && inputEndDateTime.toLocalTime().compareTo(endTime2) <= 0) {
System.out.println("属于第二个时间区间");
}
```
这样就能正确判断输入时间段是否在第二个时间区间中了。
public String addAttendance(HttpSession session) { Integer userId = (Integer) session.getAttribute("userid"); // Integer userId =1; LocalDateTime now = LocalDateTime.now(); Attendance lastAttendance = attendanceService.getByAscTime(userId); Attendance attendance = new Attendance(); attendance.setUserid(userId); attendance.setDate(Date.from(now.atZone(ZoneId.systemDefault()).toInstant())); // 获取当前本机时间 LocalDate localDate = now.toLocalDate(); LocalTime localTime = now.toLocalTime(); // 判断数据库没有打卡记录或者有下班签到 if (lastAttendance == null || lastAttendance.getType().equals("下班签到")) { if (localTime.isBefore(LocalTime.of(9, 0))) { attendance.setType("上班签到"); } else if (localDate.equals(getLastAttendanceDate(lastAttendance))) { attendance.setType("下班签到"); } else if (localTime.isAfter(LocalTime.of(9, 0))){ attendance.setType("迟到"); }else if (localTime.isBefore(LocalTime.of(9, 17))){ attendance.setType("早退"); } } else { if (localDate.equals(getLastAttendanceDate(lastAttendance))) { if (localTime.isBefore(LocalTime.of(9, 0))) { attendance.setType("上班签到"); } else if (localTime.isBefore(LocalTime.of(17, 0))){ attendance.setType("早退"); }else if (localTime.isBefore(LocalTime.of(17, 0))){ attendance.setType("下班签到"); } } else { if (localTime.isBefore(LocalTime.of(9, 0))) { attendance.setType("上班签到"); } else if (localTime.isAfter(LocalTime.of(9, 0))){ attendance.setType("迟到"); }else if (localTime.isBefore(LocalTime.of(17, 0))){ attendance.setType("早退"); } } } attendanceService.save(attendance); return "success"; } private LocalDate getLastAttendanceDate(Attendance lastAttendance) { if (lastAttendance == null) { return null; } Date date = lastAttendance.getDate(); Instant instant = date.toInstant(); ZoneId zoneId = ZoneId.systemDefault(); return instant.atZone(zoneId).toLocalDate(); } }
这段代码是一个Java方法,用于添加考勤记录。接收一个`HttpSession`对象作为参数,从中获取当前用户的id,然后根据当前时间判断考勤类型,并将考勤记录保存到数据库中。
下面是具体的解释:
- `Integer userId = (Integer) session.getAttribute("userid")`:从`HttpSession`对象中获取当前用户的id。
- `LocalDateTime now = LocalDateTime.now()`:获取当前时间。
- `Attendance lastAttendance = attendanceService.getByAscTime(userId)`:根据用户id获取最近一次考勤记录。
- `Attendance attendance = new Attendance()`:创建一个新的考勤记录。
- `attendance.setUserid(userId)`:将当前用户的id设置为考勤记录的用户id。
- `attendance.setDate(Date.from(now.atZone(ZoneId.systemDefault()).toInstant()))`:将当前时间转换成`java.util.Date`类型,并设置为考勤记录的日期。
- `if (lastAttendance == null || lastAttendance.getType().equals("下班签到"))`:判断最近一次考勤记录是否为空或者为“下班签到”。如果是,则当前考勤记录为“上班签到”或“迟到”或“早退”。如果不是,则当前考勤记录为“下班签到”或“早退”。
- `if (localTime.isBefore(LocalTime.of(9, 0)))`:判断当前时间是否早于上午9点。
- `else if (localDate.equals(getLastAttendanceDate(lastAttendance)))`:判断当前日期是否与最近一次考勤记录的日期相同。
- `else if (localTime.isAfter(LocalTime.of(9, 0)))`:判断当前时间是否晚于上午9点。
- `else if (localTime.isBefore(LocalTime.of(9, 17)))`:判断当前时间是否早于上午9点17分。
- `else if (localTime.isBefore(LocalTime.of(17, 0)))`:判断当前时间是否早于下午5点。
- `else if (localTime.isBefore(LocalTime.of(17, 0)))`:判断当前时间是否早于下午5点。
- `attendanceService.save(attendance)`:将考勤记录保存到数据库。
- `return "success"`:返回一个字符串表示操作成功。
阅读全文
相关推荐
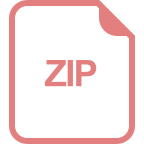
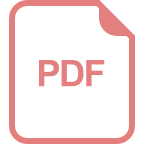


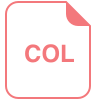











