pychme跨年烟花代码
时间: 2024-12-25 16:20:11 浏览: 23
### 使用 PyCharm 编写跨年烟花效果
为了在 PyCharm 中编写一个能够展示跨年烟花效果的 Python 程序,推荐使用 `pygame` 库。下面提供一段完整的代码示例,这段代码可以在 PyCharm 中运行并显示烟花效果。
#### 安装依赖库
首先,在 PyCharm 终端安装所需的 `pygame` 库:
```bash
pip install pygame
```
#### 导入必要的模块
接着,在项目文件中导入所需模块,并初始化游戏引擎:
```python
import random
import math
import sys
import pygame
from pygame.locals import *
```
#### 设置屏幕参数与颜色定义
配置基本的游戏窗口尺寸以及一些常用的颜色变量:
```python
# 屏幕宽度和高度设置
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
# 颜色定义 (R, G, B)
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('New Year Fireworks')
clock = pygame.time.Clock()
class Particle(pygame.sprite.Sprite):
"""粒子类用于模拟单个火花"""
def __init__(self, x, y, color=WHITE):
super().__init__()
self.x = x
self.y = y
angle = random.uniform(0, 2 * math.pi)
speed = random.uniform(1, 7)
self.vx = speed * math.cos(angle)
self.vy = -speed * math.sin(angle) # 向上发射
self.color = color
self.lifetime = 40
def update(self):
self.x += self.vx
self.y += self.vy
self.vy += 0.1 # 加重力影响使粒子下落加速
self.lifetime -= 1
if self.lifetime <= 0 or self.y >= SCREEN_HEIGHT:
self.kill() # 当寿命结束或超出边界时销毁对象
def draw(self, surface):
pygame.draw.circle(surface, self.color, (int(self.x), int(self.y)), 2)
def create_firework(x, y):
"""创建一组随机分布的新年焰火"""
colors = [(random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) for _ in range(random.randint(30, 50))]
particles_group.empty()
for i in range(len(colors)):
particle = Particle(x=x, y=y, color=colors[i])
particles_group.add(particle)
particles_group = pygame.sprite.Group()
while True:
screen.fill(BLACK)
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == MOUSEBUTTONDOWN and event.button == 1:
mouse_x, mouse_y = pygame.mouse.get_pos()
create_firework(mouse_x, mouse_y)
particles_group.update()
for p in particles_group.sprites():
p.draw(screen)
pygame.display.flip()
clock.tick(60)
```
此段代码实现了基础版本的烟花爆炸视觉效果[^1]。每当点击鼠标左键时会在当前位置触发一次烟花绽放的效果。通过调整粒子的数量、速度和其他属性可以让整个场景看起来更加绚丽多彩。
阅读全文
相关推荐
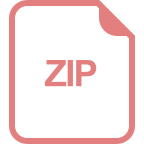
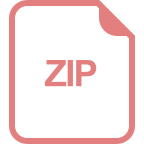
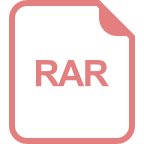
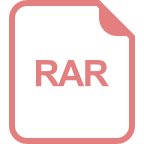
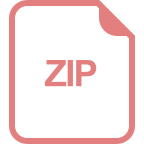
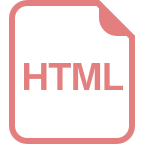
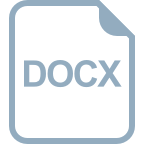
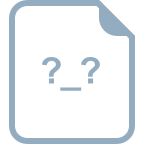
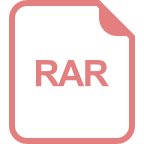
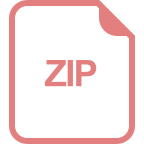
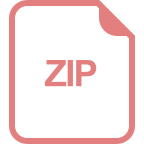
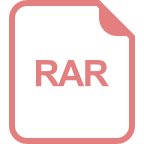
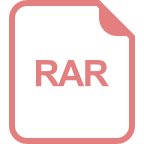
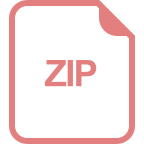
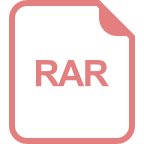
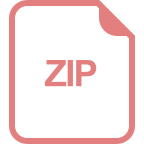