用C语言提取csv文件数据生成MD5码
时间: 2023-12-07 17:02:15 浏览: 139
好的,您可以使用C语言中的标准库和第三方库来实现这个功能。具体步骤如下:
1. 导入标准库和第三方库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/md5.h>
```
2. 使用标准库中的fopen函数打开CSV文件:
```c
FILE *fp = fopen("your_file.csv", "r");
if(fp == NULL) {
printf("Error opening file\n");
return 1;
}
```
3. 使用标准库中的fgets函数逐行读取CSV文件数据:
```c
char line[1024];
char data[1024];
while(fgets(line, 1024, fp)) {
strcat(data, line);
}
```
4. 使用第三方库中的MD5函数生成MD5码:
```c
unsigned char digest[MD5_DIGEST_LENGTH];
MD5((unsigned char *)data, strlen(data), digest);
char md5string[MD5_DIGEST_LENGTH * 2 + 1];
for(int i = 0; i < MD5_DIGEST_LENGTH; i++) {
sprintf(&md5string[i*2], "%02x", (unsigned int)digest[i]);
}
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/md5.h>
int main() {
FILE *fp = fopen("your_file.csv", "r");
if(fp == NULL) {
printf("Error opening file\n");
return 1;
}
char line[1024];
char data[1024];
while(fgets(line, 1024, fp)) {
strcat(data, line);
}
unsigned char digest[MD5_DIGEST_LENGTH];
MD5((unsigned char *)data, strlen(data), digest);
char md5string[MD5_DIGEST_LENGTH * 2 + 1];
for(int i = 0; i < MD5_DIGEST_LENGTH; i++) {
sprintf(&md5string[i*2], "%02x", (unsigned int)digest[i]);
}
printf("%s\n", md5string);
fclose(fp);
return 0;
}
```
注意,这个方法生成的MD5码是基于CSV文件中的所有数据生成的。如果您只需要对某些特定的数据生成MD5码,可以使用标准库的文件操作函数和字符串操作函数筛选数据。
阅读全文
相关推荐
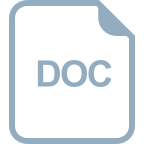
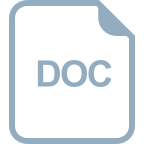
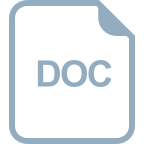
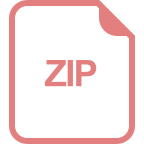

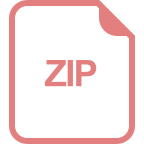
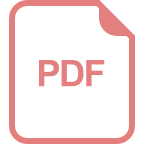
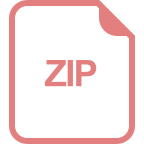

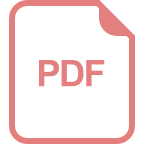
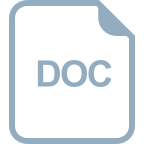
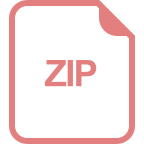


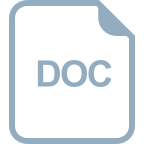