用c语言设计算法并编写程序,定义,输入(或赋随机数)和输出有100个整数元素的一堆数组,分别统计其中大于等于90,80~89,70~79,60~69,小于60的元素
时间: 2023-10-08 12:12:13 浏览: 53
以下是使用C语言的一种实现方式,与上面的Python代码相同,使用五个整型变量分别记录大于等于90,80~89,70~79,60~69,小于60的元素的个数,遍历数组并将元素按照其大小分别加入不同的计数器中,最后输出各个计数器的值即可。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
// 定义数组长度为100
const int arr_len = 100;
// 定义计数器
int counter_90_and_above = 0;
int counter_80_to_89 = 0;
int counter_70_to_79 = 0;
int counter_60_to_69 = 0;
int counter_below_60 = 0;
// 随机生成100个元素的数组
int arr[arr_len];
srand(time(NULL));
for (int i = 0; i < arr_len; i++) {
arr[i] = rand() % 101;
}
// 遍历数组并计数
for (int i = 0; i < arr_len; i++) {
if (arr[i] >= 90) {
counter_90_and_above++;
} else if (arr[i] >= 80) {
counter_80_to_89++;
} else if (arr[i] >= 70) {
counter_70_to_79++;
} else if (arr[i] >= 60) {
counter_60_to_69++;
} else {
counter_below_60++;
}
}
// 输出计数器的值
printf("大于等于90的元素有:%d\n", counter_90_and_above);
printf("80~89的元素有:%d\n", counter_80_to_89);
printf("70~79的元素有:%d\n", counter_70_to_79);
printf("60~69的元素有:%d\n", counter_60_to_69);
printf("小于60的元素有:%d\n", counter_below_60);
return 0;
}
```
注意,在实际应用中输入数据的方式可能会有所不同,此处仅提供一种生成随机数组的方法作为演示。
相关推荐
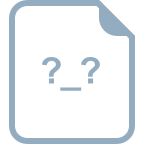
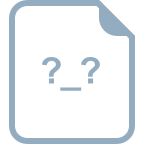
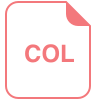
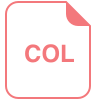
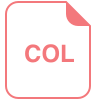











