c++提取一个文本文件中的数值,将它们写入另一个新文件
时间: 2023-05-28 08:06:29 浏览: 176
可以使用文件读写操作和正则表达式来实现。
例如,假设原始文本文件名为"input.txt",新文件名为"output.txt",可以采用以下代码:
```python
import re
# 打开文件
with open("input.txt", "r") as f1, open("output.txt", "w") as f2:
# 读取文件内容
content = f1.read()
# 查找所有数值
numbers = re.findall(r'\d+', content)
# 将数值写入新文件
for number in numbers:
f2.write(number + '\n')
```
上述代码中,首先使用`with`语句打开两个文件,分别为只读模式的原始文本文件和只写模式的新文件。然后使用`read()`方法读取原始文件的全部内容,使用正则表达式`'\d+'`查找所有数值,并使用`findall()`方法将它们保存在一个列表中。最后使用`write()`方法将每个数值写入新文件,并在每个数值后面添加一个换行符。
相关问题
使用c++语言提取一个文本文件中的数值,将它们写入另一个新文件,由空白或其他字符分开的数字被认为是不同的数值
以下是使用C语言提取文本文件中数值的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
int main() {
FILE *input_file, *output_file;
char input_filename[50], output_filename[50], c;
int num, is_num = 0;
// 打开输入文件
printf("Enter the input filename: ");
scanf("%s", input_filename);
input_file = fopen(input_filename, "r");
if (input_file == NULL) {
printf("Error opening input file.\n");
exit(1);
}
// 打开输出文件
printf("Enter the output filename: ");
scanf("%s", output_filename);
output_file = fopen(output_filename, "w");
if (output_file == NULL) {
printf("Error opening output file.\n");
exit(1);
}
// 逐个读取输入文件中的字符,并提取数字
while ((c = fgetc(input_file)) != EOF) {
if (isdigit(c)) {
is_num = 1;
num = num * 10 + (c - '0');
} else if (is_num) {
fprintf(output_file, "%d ", num);
num = 0;
is_num = 0;
}
}
// 如果最后一个字符是数字,则要将其写入输出文件中
if (is_num) {
fprintf(output_file, "%d ", num);
}
// 关闭文件
fclose(input_file);
fclose(output_file);
return 0;
}
```
该代码首先要求用户输入输入文件和输出文件的文件名,然后打开这两个文件。接着,它逐个读取输入文件中的字符,并判断这个字符是否是数字。如果是数字,则将其加入到一个整数变量中;如果不是数字且之前读取的字符中包含数字,则将这个数字写入输出文件中。最后,如果最后一个字符是数字,则将其写入输出文件中。
需要注意的是,该代码假设输入文件中每个数字之间都由空格或其他字符分开。如果有两个数字之间没有空格或其他字符,则它们将被认为是同一个数字。如果需要进一步处理这种情况,可以在代码中进行修改。
c++提取一个文本文件中的数值 将他们写入一个新文件,由空白或其他字符分开的数字被认为是不同的数值
以下是Python代码实现:
```python
with open('input.txt', 'r') as input_file:
# 读取文件中的所有内容
content = input_file.read()
# 将非数字字符替换为空格
for char in content:
if not char.isdigit():
content = content.replace(char, " ")
# 将字符串转换为数字列表
numbers_list = [int(num) for num in content.split()]
# 将数字列表写入新文件
with open('output.txt', 'w') as output_file:
for num in numbers_list:
output_file.write(str(num) + "\n")
```
以上代码将原始文件名设为“input.txt”,将提取的数字写入新文件“output.txt”。该代码首先读取原始文件中的所有内容,然后将非数字字符替换为空格。然后将字符串转换为数字列表,并将数字列表写入新文件。
阅读全文
相关推荐
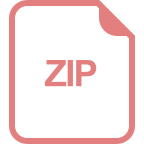
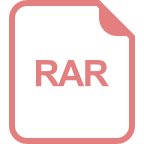
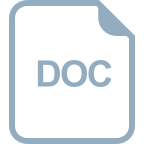
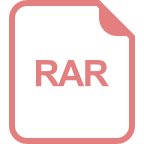
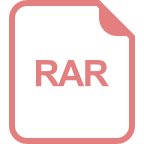
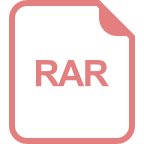
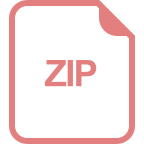
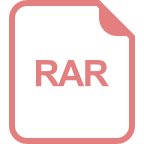
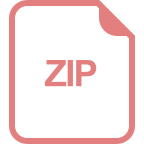
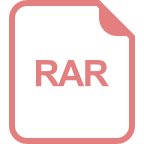
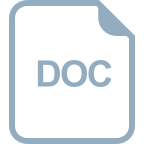
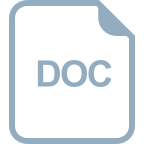
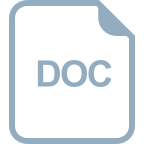
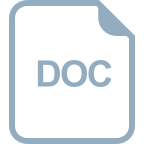