给出n个字符串,将每个字符串的大写字母修改成小写字母,小写字母修改成大写字母,然后按照字典序从小到大输出。
时间: 2024-04-30 12:23:45 浏览: 8
可以使用一个循环遍历每个字符串,然后对每个字符串中的每个字符进行判断和修改。具体步骤如下:
1. 使用一个循环遍历每个字符串:
```
for (int i = 0; i < n; i++) {
// 处理第 i 个字符串
}
```
2. 对每个字符串中的每个字符进行判断和修改:
```
for (int j = 0; j < str[i].length(); j++) {
char c = str[i][j];
if (c >= 'a' && c <= 'z') {
// 小写字母修改为大写字母
str[i][j] = c - 'a' + 'A';
} else if (c >= 'A' && c <= 'Z') {
// 大写字母修改为小写字母
str[i][j] = c - 'A' + 'a';
}
}
```
3. 最后按照字典序从小到大输出:
```
sort(str, str + n);
for (int i = 0; i < n; i++) {
cout << str[i] << endl;
}
```
完整代码如下:
```
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int n;
cin >> n;
string str[n];
for (int i = 0; i < n; i++) {
cin >> str[i];
for (int j = 0; j < str[i].length(); j++) {
char c = str[i][j];
if (c >= 'a' && c <= 'z') {
// 小写字母修改为大写字母
str[i][j] = c - 'a' + 'A';
} else if (c >= 'A' && c <= 'Z') {
// 大写字母修改为小写字母
str[i][j] = c - 'A' + 'a';
}
}
}
sort(str, str + n);
for (int i = 0; i < n; i++) {
cout << str[i] << endl;
}
return 0;
}
```
相关推荐
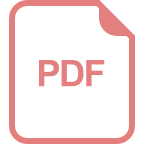
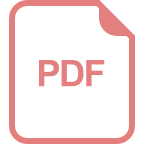
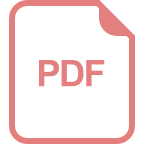














