在一个含有4层的Json数组中添加元素,并输出添加后的json
时间: 2024-03-08 20:49:07 浏览: 59
假设原始的 JSON 数组如下所示:
```
[
{
"id": 1,
"name": "John",
"age": 30,
"children": [
{
"id": 100,
"name": "Mary",
"age": 5,
"hobbies": [
"reading",
"painting"
]
}
]
},
{
"id": 2,
"name": "Jane",
"age": 35,
"children": [
{
"id": 200,
"name": "Bob",
"age": 10,
"hobbies": [
"swimming",
"dancing"
]
},
{
"id": 201,
"name": "Alice",
"age": 8,
"hobbies": [
"singing",
"playing instruments"
]
}
]
}
]
```
如果要在 `children` 数组中添加一个新的元素,可以使用以下代码:
```
// 解析 JSON 数组
JArray jsonArray = JArray.Parse(jsonString);
// 创建新的子元素
JObject newChild = new JObject();
newChild["id"] = 202;
newChild["name"] = "Tom";
newChild["age"] = 6;
// 添加新的子元素
jsonArray[1]["children"].Last.AddAfterSelf(newChild);
// 输出添加后的 JSON 数组
Console.WriteLine(jsonArray.ToString());
```
上述代码会在第二个对象中的 `children` 数组中添加一个新的元素,新元素的属性值为:
```
{
"id": 202,
"name": "Tom",
"age": 6
}
```
输出的结果为:
```
[
{
"id": 1,
"name": "John",
"age": 30,
"children": [
{
"id": 100,
"name": "Mary",
"age": 5,
"hobbies": [
"reading",
"painting"
]
}
]
},
{
"id": 2,
"name": "Jane",
"age": 35,
"children": [
{
"id": 200,
"name": "Bob",
"age": 10,
"hobbies": [
"swimming",
"dancing"
]
},
{
"id": 201,
"name": "Alice",
"age": 8,
"hobbies": [
"singing",
"playing instruments"
]
},
{
"id": 202,
"name": "Tom",
"age": 6
}
]
}
]
```
可以看到,新的元素已经成功添加到了 JSON 数组中。
阅读全文
相关推荐

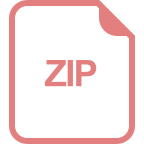
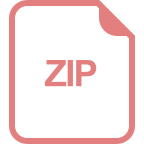
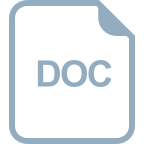
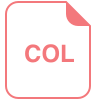
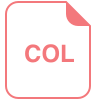
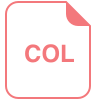
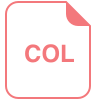
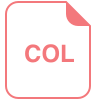
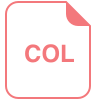
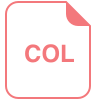
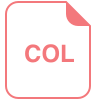
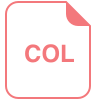
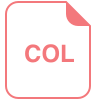
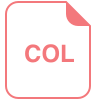
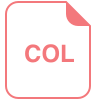