帮我写一份用C++获取图片像素点矩阵的代码
时间: 2023-05-21 18:01:21 浏览: 136
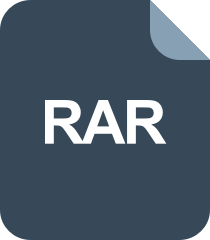
获取图片某点的像素值

以下是获取图片像素点矩阵的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#pragma pack(push, 1)
typedef struct {
uint16_t type; // 文件类型,必须是0x4D42
uint32_t size; // 文件大小
uint16_t reserved1; // 保留,必须是0
uint16_t reserved2; // 保留,必须是0
uint32_t offset; // 位图数据的起始位置
uint32_t header_size; // 位图信息头的大小,必须是40
int32_t width; // 图像宽度
int32_t height; // 图像高度
uint16_t planes; // 位图平面数,必须是1
uint16_t bits_per_pixel; // 每个像素的位数,必须是1、4、8、16、24或32
uint32_t compression; // 压缩类型,0表示不压缩
uint32_t image_size; // 图像数据大小,如果压缩类型是0,则可以设置为0
int32_t x_pixels_per_meter; // 水平分辨率,单位是像素/米
int32_t y_pixels_per_meter; // 垂直分辨率,单位是像素/米
uint32_t colors_used; // 使用的颜色数,如果每个像素的位数大于8,则可以设置为0
uint32_t colors_important; // 重要的颜色数,如果每个像素的位数大于8,则可以设置为0
} bmp_header_t;
#pragma pack(pop)
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <bmp_file>\n", argv[0]);
return 1;
}
FILE *fp = fopen(argv[1], "rb");
if (!fp) {
fprintf(stderr, "Failed to open file: %s\n", argv[1]);
return 1;
}
bmp_header_t header;
fread(&header, sizeof(header), 1, fp);
if (header.type != 0x4D42) {
fprintf(stderr, "Invalid BMP file: %s\n", argv[1]);
fclose(fp);
return 1;
}
if (header.bits_per_pixel != 24) {
fprintf(stderr, "Unsupported BMP format: %s\n", argv[1]);
fclose(fp);
return 1;
}
uint8_t *data = (uint8_t *) malloc(header.image_size);
fseek(fp, header.offset, SEEK_SET);
fread(data, header.image_size, 1, fp);
fclose(fp);
uint8_t *p = data;
for (int y = 0; y < header.height; y++) {
for (int x = 0; x < header.width; x++) {
uint8_t b = *p++;
uint8_t g = *p++;
uint8_t r = *p++;
printf("(%d, %d): %02X%02X%02X\n", x, y, r, g, b);
}
}
free(data);
return 0;
}
```
这段代码可以读取一个BMP格式的图片文件,并输出每个像素点的RGB值。如果需要处理其它格式的图片,需要修改代码中的一些细节。
阅读全文
相关推荐
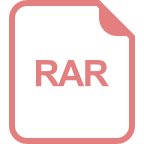
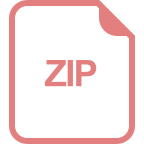
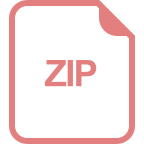
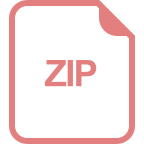
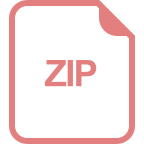
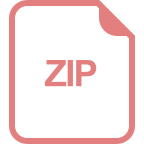
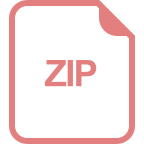
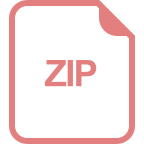
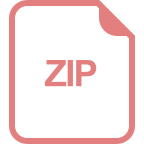
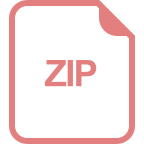
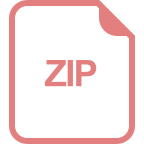
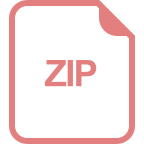