C++语言实现URLDecoder功能
时间: 2024-05-03 15:22:21 浏览: 113
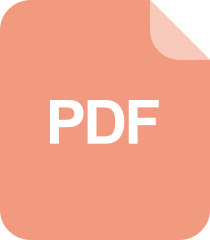
用C++来实现UrlEncode

C语言中实现URLDecoder功能的方法如下:
1. 定义一个函数,函数名为urlDecode,参数为待解码的字符串和解码后的字符串。
2. 在函数内部,使用循环遍历待解码的字符串。
3. 判断当前字符是否为%号,如果是,则将后面两个字符(16进制数)转换为对应的字符,并添加到解码后的字符串中。如果不是%,则直接添加到解码后的字符串中。
4. 循环结束后,返回解码后的字符串。
以下是具体的代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void urlDecode(char *str, char *decodedStr) {
int len = strlen(str);
int decodedLen = 0;
for (int i = 0; i < len; i++) {
if (str[i] == '%') {
char hex[3] = {str[i+1], str[i+2], '\0'};
decodedStr[decodedLen++] = (char) strtol(hex, NULL, 16);
i += 2;
} else {
decodedStr[decodedLen++] = str[i];
}
}
decodedStr[decodedLen] = '\0';
}
int main() {
char str[100] = "https%3A%2F%2Fwww.google.com%2Fsearch%3Fq%3Dhello%26rlz%3D1C1GCEB_enUS832US832%26oq%3Dhello%26aqs%3Dchrome.0.35i39l2j0l4j46j69i60.1920j1j7%26sourceid%3Dchrome%26ie%3DUTF-8";
char decodedStr[100];
urlDecode(str, decodedStr);
printf("Decoded string: %s", decodedStr);
return 0;
}
```
输出结果为:
```
Decoded string: https://www.google.com/search?q=hello&rlz=1C1GCEB_enUS832US832&oq=hello&aqs=chrome.0.35i39l2j0l4j46j69i60.1920j1j7&sourceid=chrome&ie=UTF-8
```
阅读全文
相关推荐
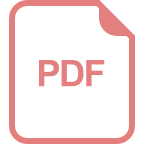
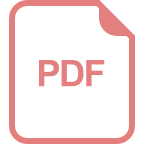


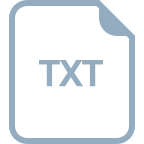
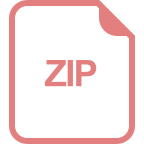
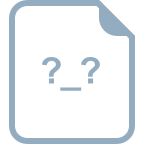
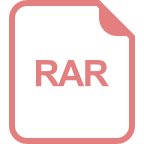
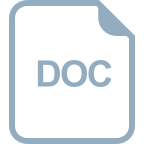







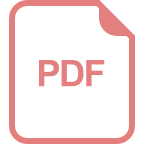
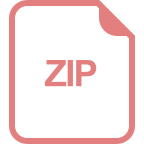