微信小程序日历实现思路
时间: 2023-09-14 13:06:15 浏览: 231
微信小程序中实现日历可以采用以下思路:
1. 实现基础的日历组件:根据当前时间,呈现出当月的日历,可以通过WXML+CSS实现。
2. 实现日历组件的交互:通过JS实现日历组件的交互,包括实现翻页功能(切换月份)、选择日期、显示特定日期的任务等。
3. 与后台数据交互:如果需要在日历上显示某个日期的任务或事件,需要与后台进行数据交互,并将数据展示在日历上。
4. 实现日历的自定义样式:可以提供不同的主题样式供用户选择,或者允许用户自行设置样式。
5. 优化性能:为了提高用户体验,需要对日历进行性能优化,如利用缓存机制、懒加载等。
总之,实现微信小程序日历需要考虑到交互、数据交互、自定义样式等方面,并进行性能优化。
相关问题
vue 微信小程序 日历
Vue 微信小程序中的日历可以通过使用第三方组件实现,如iview-weapp、vant-weapp等。
在Vue中使用微信小程序的日历组件,需要先将组件引入到项目中,并且注册为全局组件,然后在页面中使用该组件即可。
首先,将第三方组件下载并引入到项目中,然后在main.js中进行全局注册:
```js
import Vue from 'vue'
import Calendar from 'path/to/calendar'
Vue.component('calendar', Calendar)
```
然后在需要使用日历的页面中,通过标签的形式使用日历组件:
```html
<template>
<view class="container">
<calendar @choose="onChoose"></calendar>
</view>
</template>
<script>
export default {
methods: {
onChoose(date) {
console.log(date)
}
}
}
</script>
```
上述代码中,通过在template中使用`<calendar>`标签来调用日历组件,并通过`@choose`事件监听用户选择日期的操作。当用户选择日期后,会触发`onChoose`方法,将选择的日期传递给该方法。
通过上述步骤,就可以在Vue微信小程序中使用日历组件了。当然,具体的日历组件的功能和样式可以根据实际需求进行定制,以上代码只是简单示例。
总结:Vue 微信小程序中使用日历组件的步骤大致如上所述,请根据自己的项目需求来选择合适的第三方日历组件,并进行相应的引入、注册和使用。
微信小程序日历页面前端
实现一个微信小程序日历页面,可以按照以下步骤进行:
1. 创建一个新的微信小程序项目,使用微信开发者工具打开。
2. 在项目中创建一个新的页面,命名为“calendar”。
3. 在 calendar 页面中编写 HTML、CSS 和 JavaScript 代码,实现日历页面的 UI 和功能。
4. 在 HTML 中创建一个日历的容器,可以使用 table、div 等标签来创建。
5. 使用 JavaScript 生成日历的日期,可以使用 Date 对象来获取当前日期和月份,然后计算出日历中的每一天。
6. 将生成的日期填充到日历容器中,可以使用 DOM 操作来实现。
7. 实现日历的点击事件,当用户点击某一天时,可以在页面中显示该日期的详细信息。
8. 根据需要,可以在页面中添加其他功能,比如切换月份、添加事件等。
需要注意的是,在微信小程序中,需要使用小程序提供的 API 来实现一些特殊的功能,比如获取用户信息、调用微信支付等。在开发过程中,可以参考微信小程序官方文档和示例代码,以便更好地理解和使用 API。
相关推荐
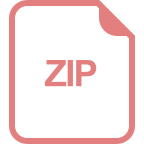
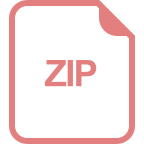












